Very often working with lots of servers in the network we would like to collect network card(s) properties of all servers on the network in an easy way. Here is where PowerShell steps forward to provide easy and fast solutions.
To get Network Card properties using PowerShell we can use either WMI or CIM classes, Win32_NetworkAdapter or CIM_NetworkAdapter respectively.
In this article will be presented to you many examples to provide you a variety of solutions.
Table of Contents
Get Network Card Properties Using PowerShell – Solutions
Here are few solutions for the local machine, remote computers, and writing own PowerShell CmdLet.
Solution 1 – Get Network Card Properties Using PowerShell For The Local Machine.
To get Network Card(s) properties for the local machine we call Get-CimInstance CmdLet and get the necessary data from CIM_NetworkAdapter CIM Class.
Get-CimInstance -Class CIM_NetworkAdapter -ComputerName localhost -ErrorAction Stop | Select-Object *
Here is the result set for the local machine:
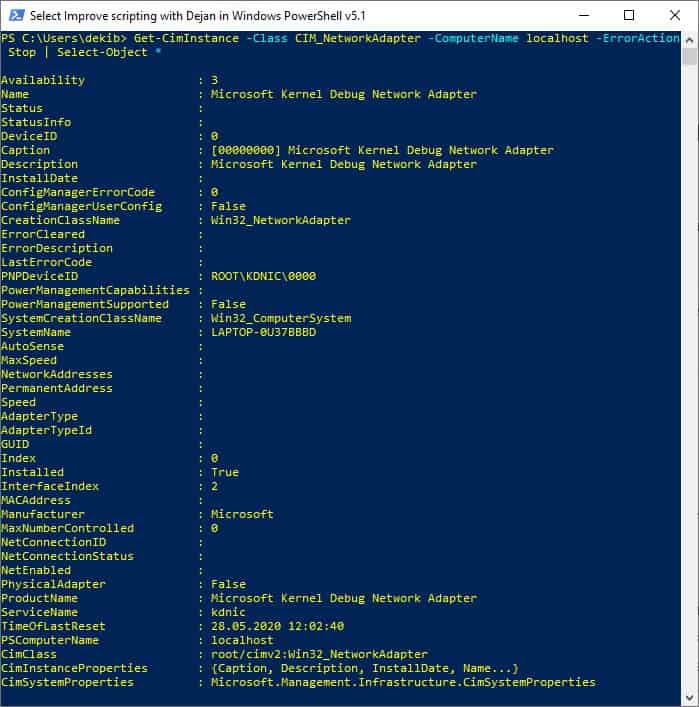
Solution 2 – Get Network Card Properties Using PowerShell For Remote Computers.
For the list of computers, we can use the same call as for the previous solution only to use the ComputerName parameter and add the list of servers as a txt file.
Create the list of servers in the text file and save in, for example, C:\Temp folder and run the same command as in the previous solution just use ComputerName parameter in addition. We basically load the content of the text file using Get-Content CmdLet and PowerShell will go through the list and run the same command as in the previous solution for each server on the list.
Get-CimInstance -Class CIM_NetworkAdapter -ComputerName (Get-Content -Path C:\Temp\servers.txt) -ErrorAction Stop | Select-Object * | Out-GridView
Here is the result set for the list of servers:

I do not have servers in my home network so in order to simulate one I have created a very simple input list of servers in a text file just copying the localhost value several times.
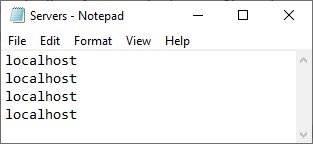
Solution 3 – Write Own PowerShell CmdLet ( Get-NetworkInfo ) To Get Network Card Properties Using PowerShell
This approach takes a little bit longer time but gives us more benefits in the long run so it is my preferred way and we can combine this CmdLet with the library of CmdLets in Efficiency Booster PowerShell Project.
I have written my own CmdLet Get-NetworkInfo CmdLet that I will explain to you shortly.
Here is one example of calling Get-NetworkInfo CmdLet:
Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose | Select-Object 'Environment', 'Logical name', 'Server name', 'Product name', 'Name', 'Manufacturer', 'Physical adapter', 'Speed', 'MAC Address', 'GUID', 'Adapter type', 'Description', 'Device ID', 'Net connection ID', 'Net connection status', 'Net enabled', 'Service name', 'Status', 'Status info', 'IP', 'Collected' | Out-GridView
Here is the result set while calling Get-NetworkInfo CmdLet:
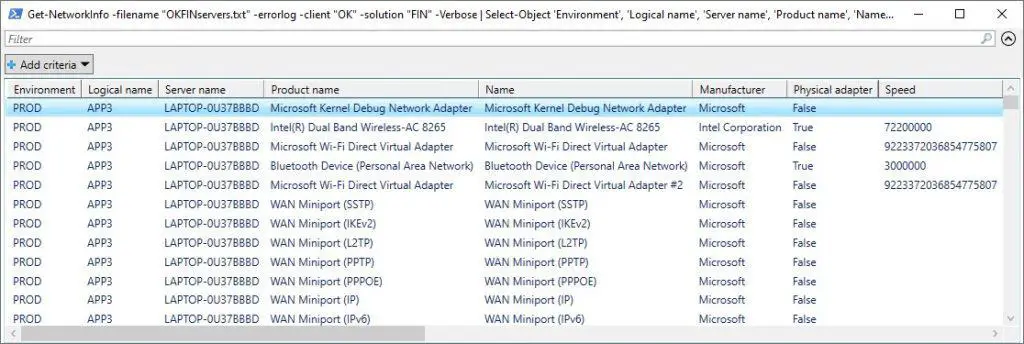
INFO: I have written two PowerShell Add-on functions ( Get-CIMClassProperty and Select-CIMClassAllProperties) that help us working with WMI and CIM classes. First list all the properties and datatypes of WMI or CIM classes and the second one makes the select statements with all the properties for the WMI or CIM class. How To List CIM Or WMI Class All Properties And Their Datatypes With PowerShell AND How To Write Select Statement For All Properties Of CIM Or WMI Class With PowerShell.
Get-NetworkInfo CmdLet Explained
Get-NetworkInfo CmdLet collects Network Card(s) Properties for the list of servers and this CmdLet belongs to Efficiency Booster PowerShell Project. This project is the library of different CmdLets that can help us IT personal to do our everyday tasks more efficiently and accurately.
Source code for Get-NetworkInfo CmdLet can be downloaded from this zip file so please feel free to download it and it would be easier for you to follow me along.
Get-NetworkInfo CmdLet is part of Common module and if you have downloaded the source code it can be found in the folder …\[My] Documents\WindowsPowerShell\Modules\03common
INFO: If you want to know how to install and configure Efficiency Booster PowerShell Project files please read the following article: How To Install And Configure PowerShell: CmdLets, Modules, Profiles.
Get-NetworkInfo CmdLet – Input Parameters
As input parameters we have:
- computers – it is a list of servers passed as input parameter with default value ‘localhost’ and accepts both pipeline options. Parameter belongs to the “ServerNames” parameter set. The “ServerNames” parameter set is the default parameter set.
- filename – it is the name of the text file with the list of servers and represents an alternative option to the “computers” parameter. The parameter belongs to the “FileName” parameter set.
- errorlog – switch datatype and when turned on it will write errors into an external error log file using Write-ErrorLog CmdLet. The error log file is located in the PSLogs folder of [My] Documents.
- client – it is a mandatory input parameter and by convention, I use two letters for client shortcode (for example, OK = O client, BK = B client, etc.). This parameter value is part of the filename parameter naming convention.
- solution – it is a mandatory input parameter and by convention, I use two-three letters for solution shortcode (for example, FIN = Financial solution, HR = HR solution, etc.).
The naming convention for the filename parameter is as follows: Client + Solution + Text.txt. The text file should be located in …[My] Documents\WindowsPowerShell\Modules\01servers folder.
For example:
- OKFINTestServers.txt – List of test environment servers for OK client and FIN solution
- OKFINProdServers.txt – List of production environmentservers for OK client and FIN solution.
- OKFINAllServers.txt – List of all servers for OK client and FIN solution.
INFO: To get a deeper explanation about client and solution input parameters please read these two sections Parameter client, Parameter solution.
INFO: In order to customize installation of CmdLet to your needs and setup necessary CSV file please read the following article How To Install And Configure PowerShell: CmdLets, Modules, Profiles
Here is the parameters definition code:
Function Get-NetworkInfo {
[CmdletBinding(DefaultParametersetName="ServerNames")]
param (
[Parameter( ValueFromPipeline=$true,
ValueFromPipelineByPropertyName=$true,
ParameterSetName="ServerNames",
HelpMessage="List of computer names separated by commas.")]
[Alias('hosts')]
[string[]]$computers = 'localhost',
[Parameter( ParameterSetName="FileName",
HelpMessage="Name of txt file with list of servers. Txt file should be in 01servers folder.")]
[string]$filename,
[Parameter( Mandatory=$false,
HelpMessage="Write to error log file or not.")]
[switch]$errorlog,
[Parameter(Mandatory=$true,
HelpMessage="Client for example OK = O client, BK = B client")]
[string]$client,
[Parameter(Mandatory=$true,
HelpMessage="Solution, for example FIN = Financial, HR = Human Resource")]
[string]$solution
)
}
INFO: To know more about PowerShell Parameters and Parameter Sets with some awesome examples please read the following articles How To Create Parameters In PowerShell and How To Use Parameter Sets In PowerShell Functions.
INFO: PowerShell Pipelining is a very important concept and I highly recommend you to read the article written on the subject. How PowerShell Pipeline Works. Here I have shown in many examples the real power of PowerShell using the Pipelining.
BEGIN Block
In the BEGIN block we:
- If the FileName parameter set has been used we test if text file with a list of servers exists.
- If the file exists read the file and create the list of servers as a string array in the $computers variable.
- … and if not write a warning with information to the caller to create the file.
BEGIN {
if ( $PsCmdlet.ParameterSetName -eq "FileName") {
if ( Test-Path -Path "$home\Documents\WindowsPowerShell\Modules\01servers\$filename" -PathType Leaf ) {
Write-Verbose "Read content from file: $filename"
$computers = Get-Content( "$home\Documents\WindowsPowerShell\Modules\01servers\$filename" )
} else {
Write-Warning "This file path does NOT exist: $home\Documents\WindowsPowerShell\Modules\01servers\$filename"
Write-Warning "Create file $filename in folder $home\Documents\WindowsPowerShell\Modules\01servers with list of server names."
break;
}
}
}
PROCESS Block
In the PROCESS block we run this block of code for each server passed into the pipeline or read from the text file:
- We replace the localhost default value for the local server with the actual name of the local machine.
- We use Get-ComputerInfo CmdLet to read additional data about each server (name, environment, logical name, IP address).
- We implement Error Handling using try-catch blocks and write errors in an external text file using Write-ErrorLog CmdLet.
- We use PowerShell splatting to prepare the input parameters for the next call of Get-CimInstance CmdLet.
- We call the CIM class CIM_NetworkAdapter using Get-CimInstance CmdLet.
- We go through each Network card installed of each server and prepare the resultset of Get-NetworkInfo CmdLet.
In the PROCESS block, we would like to emphasize two things used in the code:
1 – Splatting
Here is splatting:
$params = @{ 'ComputerName'=$computer;
'Class'='CIM_NetworkAdapter';
'ErrorAction'='Stop'}
$networks = Get-CimInstance @params |
Select-Object @{label="ServerName"; Expression={$_.SystemName}},
ProductName,
Name,
Manufacturer,
PhysicalAdapter,
Speed,
MACAddress,
GUID,
AdapterType,
Description,
DeviceID,
NetConnectionID,
NetConnectionStatus,
NetEnabled,
ServiceName,
Status,
StatusInfo
2 – Result set Creation And Type Name Of Result set
Again for creation of result set we use splatting as you can see in the source code:
$properties = @{ 'Environment'=$env;
'Logical name'=$logicalname;
'Server name'=$network.ServerName;
"Product name"=$network.ProductName;
'Name'=$network.Name;
'Manufacturer'=$network.Manufacturer;
'Physical adapter'=$network.PhysicalAdapter;
'Speed'=$network.Speed;
'MAC Address'=$network.MACAddress;
'GUID'=$network.GUID;
'Adapter type'=$network.AdapterType;
'Description'=$network.Description;
'Device ID'=$network.DeviceID;
'Net connection ID'=$network.NetConnectionID;
'Net connection status'=$network.NetConnectionStatus;
'Net enabled'=$network.NetEnabled;
'Service name'=$network.ServiceName;
'Status'=$network.Status;
'Status info'=$network.StatusInfo;
'IP'=$ip;
'Collected'=(Get-Date -UFormat %Y.%m.%d' '%H:%M:%S)}
$obj = New-Object -TypeName PSObject -Property $properties
In addition, we give a name (Report.NetworkCard) to the type of result set as you can see in the code:
$obj.PSObject.TypeNames.Insert(0,'Report.NetworkCard')
So if we call PowerShell Get-Member CmdLet to get the type of result set for Get-NetworkInfo CmdLet we will get Report.NetworkCard type and not standard PowerShell PSObject type.
Get-NetworkInfo -client OK -solution FIN | Get-Member
Here is the result:
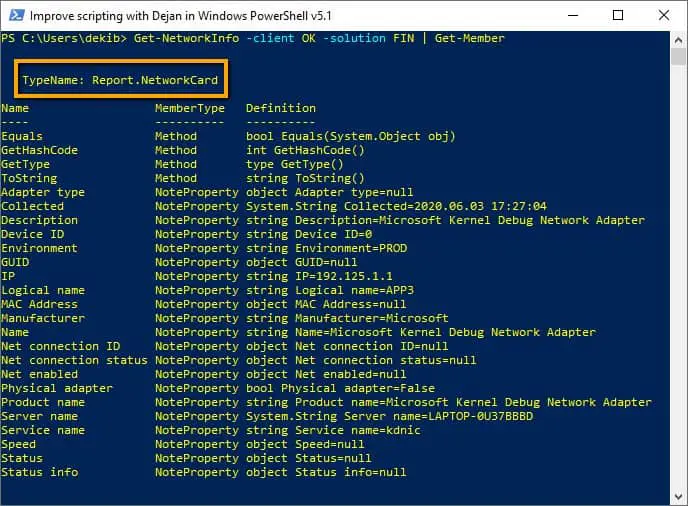
Type Name of result set gives us a possibility to further expend the use of the result set. For example, we can use MS SQL Database and create table NerworkCard based on result set type name (Report.NerworkCard) where we can record the result set and use MS SQL Reporting Services to show the results collected over a certain period of time.
Finally, here is the PROCESS block source code:
PROCESS {
foreach ($computer in $computers ) {
if ( $computer -eq 'localhost' ) {
$computer = $env:COMPUTERNAME
}
$computerinfo = Get-ComputerInfo -computername $computer -client $client -solution $solution
$hostname = $computerinfo.hostname
$env = $computerinfo.environment
$logicalname = $computerinfo.logicalname
$ip = $computerinfo.ipaddress
try {
Write-Verbose "Start processing: $computer - $env - $logicalname"
Write-Verbose "Start CIM_NetworkAdapter processing..."
# I have to null the values in order not to send network info of wrong server into error log.
$networks = $null
$obj = $null
$network = $null
$params = @{ 'ComputerName'=$computer;
'Class'='CIM_NetworkAdapter';
'ErrorAction'='Stop'}
$networks = Get-CimInstance @params |
Select-Object @{label="ServerName"; Expression={$_.SystemName}},
ProductName,
Name,
Manufacturer,
PhysicalAdapter,
Speed,
MACAddress,
GUID,
AdapterType,
Description,
DeviceID,
NetConnectionID,
NetConnectionStatus,
NetEnabled,
ServiceName,
Status,
StatusInfo
Write-Verbose "Finish CIM_NetworkAdapter processing..."
foreach ($network in $networks) {
Write-Verbose "Start processing NIC: $network"
$properties = @{ 'Environment'=$env;
'Logical name'=$logicalname;
'Server name'=$network.ServerName;
"Product name"=$network.ProductName;
'Name'=$network.Name;
'Manufacturer'=$network.Manufacturer;
'Physical adapter'=$network.PhysicalAdapter;
'Speed'=$network.Speed;
'MAC Address'=$network.MACAddress;
'GUID'=$network.GUID;
'Adapter type'=$network.AdapterType;
'Description'=$network.Description;
'Device ID'=$network.DeviceID;
'Net connection ID'=$network.NetConnectionID;
'Net connection status'=$network.NetConnectionStatus;
'Net enabled'=$network.NetEnabled;
'Service name'=$network.ServiceName;
'Status'=$network.Status;
'Status info'=$network.StatusInfo;
'IP'=$ip;
'Collected'=(Get-Date -UFormat %Y.%m.%d' '%H:%M:%S)}
$obj = New-Object -TypeName PSObject -Property $properties
$obj.PSObject.TypeNames.Insert(0,'Report.NetworkCard')
Write-Output $obj
Write-Verbose "Finish processing NIC: $network"
}
Write-Verbose "Finish processing: $computer - $env - $logicalname"
} catch {
Write-Warning "Computer failed: $computer - $env - $logicalname NIC failed: $network"
Write-Warning "Error message: $_"
if ( $errorlog ) {
$errormsg = $_.ToString()
$exception = $_.Exception
$stacktrace = $_.ScriptStackTrace
$failingline = $_.InvocationInfo.Line
$positionmsg = $_.InvocationInfo.PositionMessage
$pscommandpath = $_.InvocationInfo.PSCommandPath
$failinglinenumber = $_.InvocationInfo.ScriptLineNumber
$scriptname = $_.InvocationInfo.ScriptName
Write-Verbose "Start writing to Error log."
Write-ErrorLog -hostname $computer -env $env -logicalname $logicalname -errormsg $errormsg -exception $exception -scriptname $scriptname -failinglinenumber $failinglinenumber -failingline $failingline -pscommandpath $pscommandpath -positionmsg $pscommandpath -stacktrace $stacktrace
Write-Verbose "Finish writing to Error log."
}
}
}
}
INFO: To learn about PowerShell Error Handling and code debugging please read the following articles: How To Log PowerShell Errors And Much More and How To Debug PowerShell Scripts.
END Block
END block is empty.
INFO: To understand BEGIN, PROCESS and END blocks in PowerShell please read PowerShell Function Begin Process End Blocks Explained With Examples.
Comment-Based Help Section
For every one of my own CmdLets, I write Comment-Based help as well.
INFO: If you want to learn how to write comment-based Help for your own PowerShell Functions and Scripts please read these articles How To Write PowerShell Help (Step by Step). In this article How To Write PowerShell Function’s Or CmdLet’s Help (Fast), I explain the PowerShell Add-on that helps us to be fast with writing help content.
How To Use Get-NetworkInfo CmdLet – Tips
To get Network Cards properties for the local machine we just call Get-NetworkInfo CmdLet and provide values for mandatory parameters (client and solution):
Get-NetworkInfo -client "OK" -solution "FIN"
Here is the result set:
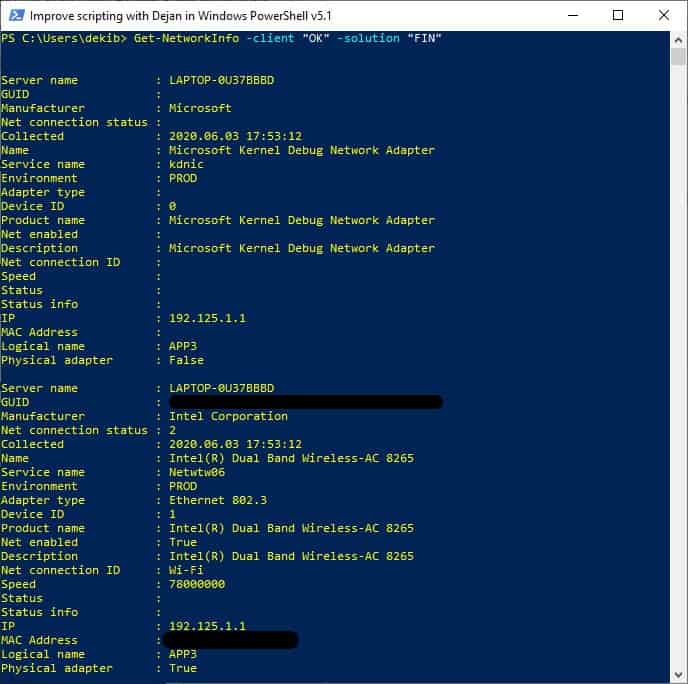
As we have seen in the solution section of this article we can call Get-NetworkInfo CmdLet for the list of servers:
Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose | Select-Object 'Environment', 'Logical name', 'Server name', 'Product name', 'Name', 'Manufacturer', 'Physical adapter', 'Speed', 'MAC Address', 'GUID', 'Adapter type', 'Description', 'Device ID', 'Net connection ID', 'Net connection status', 'Net enabled', 'Service name', 'Status', 'Status info', 'IP', 'Collected' | Out-GridView
Here is the result set for the list of servers:
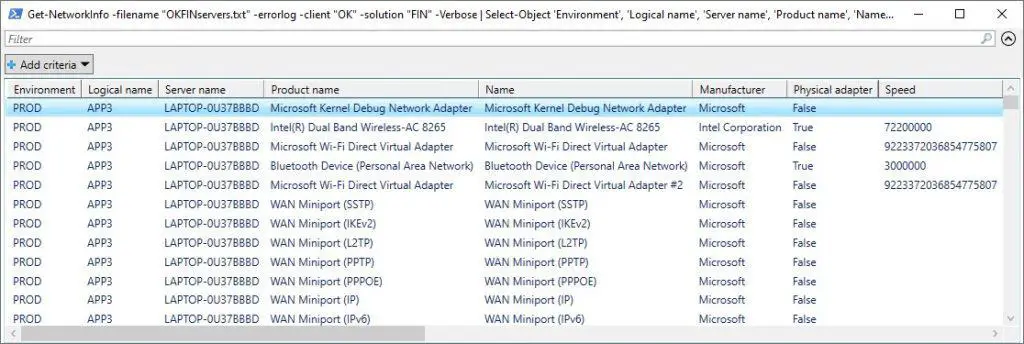
We can PowerShell pipeline result from Get-NetworkInfo CmdLet into Save-ToExcel CmdLet and get the result set as an Excel Sheet:
Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose | Select-Object 'Environment', 'Logical name', 'Server name', 'Product name', 'Name', 'Manufacturer', 'Physical adapter', 'Speed', 'MAC Address', 'GUID', 'Adapter type', 'Description', 'Device ID', 'Net connection ID', 'Net connection status', 'Net enabled', 'Service name', 'Status', 'Status info', 'IP', 'Collected' | Save-ToExcel -errorlog -ExcelFileName "Get-NetworkInfo" -title "Get Network adapters info of servers in Financial solution for " -author "Dejan Mladenovic" -WorkSheetName "Network adapters Info" -client "OK" -solution "FIN"
Here is the Excel Sheet result:
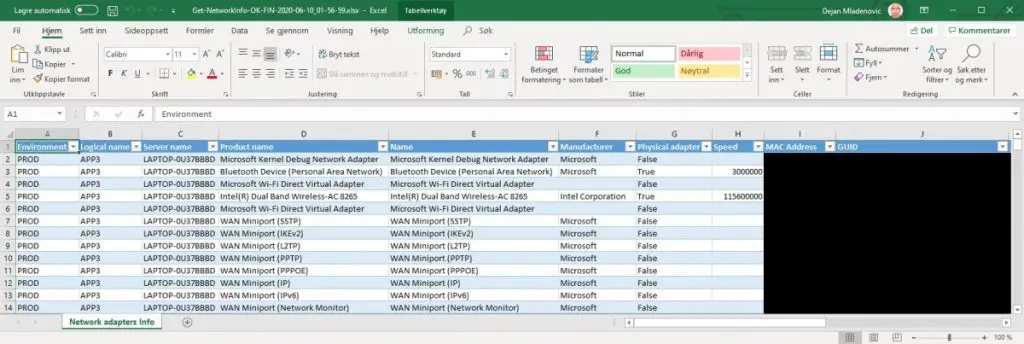
Bonus Tip: I have used Get-NetworkInfo CmdLet for documentation purposes while making a PDF document for the client where we have installed our solution.
Useful PowerShell Network Cards (Adapter) Properties Information Articles
Here are some useful articles and resources:
Get-NetworkInfo CmdLet Source Code
DISCLAIMER: Get-NetworkInfo function is part of the Efficiency Booster PowerShell Project and as such utilize other CmdLets that are part of the same project. So the best option for you in order for this function to work without any additional customization is to download the source code of the whole project from here.
INFO: My best advice to every PowerShell scripter is to learn writing own PowerShell Advanced Functions and CmdLets and I have written several articles explaining this, so please read them. How To Create A Custom PowerShell CmdLet (Step By Step). Here I explain how to use PowerShell Add-on Function to be faster in writing PowerShell Functions How To Write Advanced Functions Or CmdLets With PowerShell (Fast).
Here is the source code of the whole Get-NetworkInfo CmdLet:
<#
.SYNOPSIS
Get Network's Adapter info for list of computers.
.DESCRIPTION
Get Network's Adapters info for list of servers.
List of servers is in txt file in 01servers folder or list of strings with names of computers.
CmdLet has two ParameterSets one for list of computers from file and another from list of strings as computer names.
Errors will be saved in log folder PSLogs with name Error_Log.txt. Parameter errorlog controls logging of errors in log file.
Get-NetworkInfo function uses Get-CimInstance -Class CIM_NetworkAdapter PowerShell function to get Network Adapter info.
Result shows following columns: Environment (PROD, Acceptance, Test, Course...),
LogicalName (Application, web, integration, FTP, Scan, Terminal Server...), Server name,
Product name, Name, Manufacturer, Physical adapter, Speed, MAC Address, GUID, Adapter type, Description, Device ID,
Net connection ID, Net connection status, Net enabled, Service name, Status, Status info, IP, Collected.
.PARAMETER computers
List of computers that we want to get Network's adapters Info from. Parameter belongs to default Parameter Set = ServerNames.
.PARAMETER filename
Name of txt file with list of servers that we want to check Network's adapters info. .txt file should be in 01servers folder.
Parameter belongs to Parameter Set = FileName.
.PARAMETER errorlog
Switch parameter that sets to write to log or not to write to log. Error file is in PSLog folder with name Error_Log.txt.
.PARAMETER client
OK - O client
BK - B client
etc.
.PARAMETER solution
FIN - Financial solution
HR - Human resource solution
etc.
.EXAMPLE
Get-NetworkInfo -client "OK" -solution "FIN"
Description
---------------------------------------
Test of default parameter with default value ( computers = 'localhost' ) in default ParameterSet = ServerName.
.EXAMPLE
Get-NetworkInfo -client "OK" -solution "FIN" -Verbose
Description
---------------------------------------
Test of Verbose parameter. NOTE: Notice how localhost default value of parameter computers replaces with name of server.
.EXAMPLE
'ERROR' | Get-NetworkInfo -client "OK" -solution "FIN" -errorlog
Description
---------------------------------------
Test of errorlog parameter. There is no server with name ERROR so this call will fail and write to Error log since errorlog switch parameter is on. Look Error_Log.txt file in PSLogs folder.
.EXAMPLE
Get-NetworkInfo -computers 'APP100001' -client "OK" -solution "FIN" -errorlog
Description
---------------------------------------
Test of computers parameter with one value. Parameter accepts array of strings.
.EXAMPLE
Get-NetworkInfo -computers 'APP100001', 'APP100002' -client "OK" -solution "FIN" -errorlog -Verbose
Description
---------------------------------------
Test of computers parameter with array of strings. Parameter accepts array of strings.
.EXAMPLE
Get-NetworkInfo -hosts 'APP100001' -client "OK" -solution "FIN" -errorlog
Description
---------------------------------------
Test of computers paramater alias hosts.
.EXAMPLE
Get-NetworkInfo -computers (Get-Content( "$home\Documents\WindowsPowerShell\Modules\01servers\OKFINservers.txt" )) -client "OK" -solution "FIN" -errorlog -Verbose
Description
---------------------------------------
Test of computers parameter and values for parameter comes from .txt file that has list of servers.
.EXAMPLE
'APP100001' | Get-NetworkInfo -client "OK" -solution "FIN" -errorlog
Description
---------------------------------------
Test of pipeline by value of computers parameter.
.EXAMPLE
'APP100001', 'APP100002' | Get-NetworkInfo -client "OK" -solution "FIN" -errorlog -Verbose
Description
---------------------------------------
Test of pipeline by value with array of strings of computers parameter.
.EXAMPLE
'APP100001', 'APP100002' | Select-Object @{label="computers";expression={$_}} | Get-NetworkInfo -client "OK" -solution "FIN" -errorlog
Description
---------------------------------------
Test of values from pipeline by property name (computers).
.EXAMPLE
Get-Content( "$home\Documents\WindowsPowerShell\Modules\01servers\OKFINservers.txt" ) | Get-NetworkInfo -client "OK" -solution "FIN" -errorlog -Verbose
Description
---------------------------------------
Test of pipeline by value that comes as content of .txt file with list of servers.
.EXAMPLE
Help Get-NetworkInfo -Full
Description
---------------------------------------
Test of Powershell help.
.EXAMPLE
Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose
Description
---------------------------------------
This is test of ParameterSet = FileName and parameter filename. There is list of servers in .txt file.
.EXAMPLE
Get-NetworkInfo -filename "OKFINserverss.txt" -errorlog -client "OK" -solution "FIN" -Verbose
Description
---------------------------------------
This is test of ParameterSet = FileName and parameter filename. This test will fail due to wrong name of the .txt file with warning message "WARNING: This file path does NOT exist:".
.INPUTS
System.String
Computers parameter pipeline both by Value and by Property Name value and has default value of localhost. (Parameter Set = ComputerNames)
Filename parameter does not pipeline and does not have default value. (Parameter Set = FileName)
.OUTPUTS
System.Management.Automation.PSCustomObject
Get-NetworkInfo returns PSCustomObjects which has been converted from PowerShell function Get-CimInstance -Class CIM_NetworkAdapter
Result shows following columns: Environment (PROD, Acceptance, Test, Course...),
LogicalName (Application, web, integration, FTP, Scan, Terminal Server...), ServerName,
Product name, Name, Manufacturer, Physical adapter, Speed, MAC Address, GUID, Adapter type, Description, Device ID,
Net connection ID, Net connection status, Net enabled, Service name, Status, Status info, IP, Collected
.NOTES
FunctionName : Get-NetworkInfo
Created by : Dejan Mladenovic
Date Coded : 10/31/2018 19:06:41
More info : http://improvescripting.com/
.LINK
How To Get Network Card Properties Using PowerShell
Get-WmiObject -Class Win32_NetworkAdapter
Get-CimInstance -Class CIM_NetworkAdapter
#>
Function Get-NetworkInfo {
[CmdletBinding(DefaultParametersetName="ServerNames")]
param (
[Parameter( ValueFromPipeline=$true,
ValueFromPipelineByPropertyName=$true,
ParameterSetName="ServerNames",
HelpMessage="List of computer names separated by commas.")]
[Alias('hosts')]
[string[]]$computers = 'localhost',
[Parameter( ParameterSetName="FileName",
HelpMessage="Name of txt file with list of servers. Txt file should be in 01servers folder.")]
[string]$filename,
[Parameter( Mandatory=$false,
HelpMessage="Write to error log file or not.")]
[switch]$errorlog,
[Parameter(Mandatory=$true,
HelpMessage="Client for example OK = O client, BK = B client")]
[string]$client,
[Parameter(Mandatory=$true,
HelpMessage="Solution, for example FIN = Financial, HR = Human Resource")]
[string]$solution
)
BEGIN {
if ( $PsCmdlet.ParameterSetName -eq "FileName") {
if ( Test-Path -Path "$home\Documents\WindowsPowerShell\Modules\01servers\$filename" -PathType Leaf ) {
Write-Verbose "Read content from file: $filename"
$computers = Get-Content( "$home\Documents\WindowsPowerShell\Modules\01servers\$filename" )
} else {
Write-Warning "This file path does NOT exist: $home\Documents\WindowsPowerShell\Modules\01servers\$filename"
Write-Warning "Create file $filename in folder $home\Documents\WindowsPowerShell\Modules\01servers with list of server names."
break;
}
}
}
PROCESS {
foreach ($computer in $computers ) {
if ( $computer -eq 'localhost' ) {
$computer = $env:COMPUTERNAME
}
$computerinfo = Get-ComputerInfo -computername $computer -client $client -solution $solution
$hostname = $computerinfo.hostname
$env = $computerinfo.environment
$logicalname = $computerinfo.logicalname
$ip = $computerinfo.ipaddress
try {
Write-Verbose "Start processing: $computer - $env - $logicalname"
Write-Verbose "Start CIM_NetworkAdapter processing..."
# I have to null the values in order not to send network info of wrong server into error log.
$networks = $null
$obj = $null
$network = $null
$params = @{ 'ComputerName'=$computer;
'Class'='CIM_NetworkAdapter';
'ErrorAction'='Stop'}
$networks = Get-CimInstance @params |
Select-Object @{label="ServerName"; Expression={$_.SystemName}},
ProductName,
Name,
Manufacturer,
PhysicalAdapter,
Speed,
MACAddress,
GUID,
AdapterType,
Description,
DeviceID,
NetConnectionID,
NetConnectionStatus,
NetEnabled,
ServiceName,
Status,
StatusInfo
Write-Verbose "Finish CIM_NetworkAdapter processing..."
foreach ($network in $networks) {
Write-Verbose "Start processing NIC: $network"
$properties = @{ 'Environment'=$env;
'Logical name'=$logicalname;
'Server name'=$network.ServerName;
"Product name"=$network.ProductName;
'Name'=$network.Name;
'Manufacturer'=$network.Manufacturer;
'Physical adapter'=$network.PhysicalAdapter;
'Speed'=$network.Speed;
'MAC Address'=$network.MACAddress;
'GUID'=$network.GUID;
'Adapter type'=$network.AdapterType;
'Description'=$network.Description;
'Device ID'=$network.DeviceID;
'Net connection ID'=$network.NetConnectionID;
'Net connection status'=$network.NetConnectionStatus;
'Net enabled'=$network.NetEnabled;
'Service name'=$network.ServiceName;
'Status'=$network.Status;
'Status info'=$network.StatusInfo;
'IP'=$ip;
'Collected'=(Get-Date -UFormat %Y.%m.%d' '%H:%M:%S)}
$obj = New-Object -TypeName PSObject -Property $properties
$obj.PSObject.TypeNames.Insert(0,'Report.NetworkCard')
Write-Output $obj
Write-Verbose "Finish processing NIC: $network"
}
Write-Verbose "Finish processing: $computer - $env - $logicalname"
} catch {
Write-Warning "Computer failed: $computer - $env - $logicalname NIC failed: $network"
Write-Warning "Error message: $_"
if ( $errorlog ) {
$errormsg = $_.ToString()
$exception = $_.Exception
$stacktrace = $_.ScriptStackTrace
$failingline = $_.InvocationInfo.Line
$positionmsg = $_.InvocationInfo.PositionMessage
$pscommandpath = $_.InvocationInfo.PSCommandPath
$failinglinenumber = $_.InvocationInfo.ScriptLineNumber
$scriptname = $_.InvocationInfo.ScriptName
Write-Verbose "Start writing to Error log."
Write-ErrorLog -hostname $computer -env $env -logicalname $logicalname -errormsg $errormsg -exception $exception -scriptname $scriptname -failinglinenumber $failinglinenumber -failingline $failingline -pscommandpath $pscommandpath -positionmsg $pscommandpath -stacktrace $stacktrace
Write-Verbose "Finish writing to Error log."
}
}
}
}
END {
}
}
#region Execution examples
#Get-NetworkInfo -client "OK" -solution "FIN"
#Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose | Out-GridView
#Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose | Select-Object 'Environment', 'Logical name', 'Server name', 'Product name', 'Name', 'Manufacturer', 'Physical adapter', 'Speed', 'MAC Address', 'GUID', 'Adapter type', 'Description', 'Device ID', 'Net connection ID', 'Net connection status', 'Net enabled', 'Service name', 'Status', 'Status info', 'IP', 'Collected'
<#
#Test ParameterSet = ServerName
Get-NetworkInfo -client "OK" -solution "FIN"
Get-NetworkInfo -client "OK" -solution "FIN" -errorlog
Get-NetworkInfo -client "OK" -solution "FIN" -errorlog -Verbose
Get-NetworkInfo -computers 'APP100001' -client "OK" -solution "FIN" -errorlog
Get-NetworkInfo -computers 'APP100001', 'APP100002' -client "OK" -solution "FIN" -errorlog -Verbose
Get-NetworkInfo -hosts 'APP100001' -client "OK" -solution "FIN" -errorlog
Get-NetworkInfo -computers (Get-Content( "$home\Documents\WindowsPowerShell\Modules\01servers\OKFINservers.txt" )) -client "OK" -solution "FIN" -errorlog -Verbose
#Pipeline examples
'APP100001' | Get-NetworkInfo -client "OK" -solution "FIN" -errorlog
'APP100001', 'APP100002' | Get-NetworkInfo -client "OK" -solution "FIN" -errorlog -Verbose
'APP100001', 'APP100002' | Select-Object @{label="computers";expression={$_}} | Get-NetworkInfo -client "OK" -solution "FIN" -errorlog
Get-Content( "$home\Documents\WindowsPowerShell\Modules\01servers\OKFINservers.txt" ) | Get-NetworkInfo -client "OK" -solution "FIN" -errorlog -Verbose
'ERROR' | Get-NetworkInfo -client "OK" -solution "FIN" -errorlog
#Test CmdLet help
Help Get-NetworkInfo -Full
#SaveToExcel
Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose | Select-Object 'Environment', 'Logical name', 'Server name', 'Product name', 'Name', 'Manufacturer', 'Physical adapter', 'Speed', 'MAC Address', 'GUID', 'Adapter type', 'Description', 'Device ID', 'Net connection ID', 'Net connection status', 'Net enabled', 'Service name', 'Status', 'Status info', 'IP', 'Collected' | Save-ToExcel -errorlog -ExcelFileName "Get-NetworkInfo" -title "Get Network adapters info of servers in Financial solution for " -author "Dejan Mladenovic" -WorkSheetName "Network adapters Info" -client "OK" -solution "FIN"
#SaveToExcel and send email
Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose | Select-Object 'Environment', 'Logical name', 'Server name', 'Product name', 'Name', 'Manufacturer', 'Physical adapter', 'Speed', 'MAC Address', 'GUID', 'Adapter type', 'Description', 'Device ID', 'Net connection ID', 'Net connection status', 'Net enabled', 'Service name', 'Status', 'Status info', 'IP', 'Collected' | Save-ToExcel -sendemail -errorlog -ExcelFileName "Get-NetworkInfo" -title "Get Network adapters info of servers in Financial solution for " -author "Dejan Mladenovic" -WorkSheetName "Network adapter Info" -client "OK" -solution "FIN"
#Benchmark
#Time = 52; Total Items = 46
Measure-BenchmarksCmdLet { Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose }
#Time = 52 sec; Total Items = 46
Measure-BenchmarksCmdLet { Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" }
#Baseline create
Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose | Save-Baseline -errorlog -BaselineFileName "Get-NetworkInfo" -client "OK" -solution "FIN" -Verbose
#Baseline archive and create new
Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose | Save-Baseline -archive -errorlog -BaselineFileName "Get-NetworkInfo" -client "OK" -solution "FIN" -Verbose
#Test ParameterSet = FileName
Get-NetworkInfo -filename "OKFINservers.txt" -errorlog -client "OK" -solution "FIN" -Verbose
Get-NetworkInfo -filename "OKFINserverss.txt" -errorlog -client "OK" -solution "FIN" -Verbose
#>
#endregion
REMEMBER: PowerShell every day!