PowerShell can be tremendously helpful while working and manipulating Windows Services and in addition can save us a tone of time since can do the changes on remote computers as well.
In this article, we will cover all the options and how you can handle Windows Services using PowerShell CmdLets and Classes such as: Get Windows Services properties, Add, Delete, Start, Stop, Restart, Pause, Resume and Change (Set) both on the local machine and remote computers.
Table of Contents
How To Get Windows Service Properties Using PowerShell With Easy Steps
We have many different approaches to get Windows Service properties using PowerShell and we will cover as many as we can. So let’s dive into the steps:
- Open PowerShell Console running as Administrator. We can get properties of Windows Service without being Admin on the machine but since there is a high probability that we will in the next step do some other manipulation of windows service (start, stop, pause, resume, etc.). It is smart to already open console as Administrator since the start, stop pause, resume demands Admin privileges.
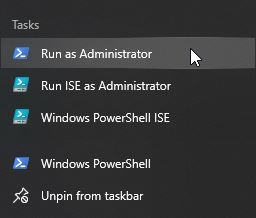
Now we can cover several calls that will return Windows Service Properties.
- We can use native PowerShell CmdLet Get-Service and provide the name of Windows Service as an input parameter (Telephony Windows Service with the name TapiSrv) that will return default properties of Windows Service (Status, Name, Display Name)
Get-Service -Name TapiSrv
We can see on the screenshot below the result of running above line of code.
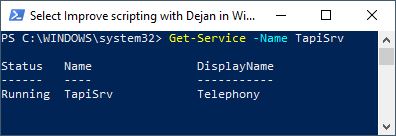
- If we want to get all the properties that return Get-Service PowerShell CmdLet we can PowerShell Pipeline the result of Get-Service to Select-Object CmdLet like in the next example.
Get-Service -Name TapiSrv | Select-Object *
Now we can see a lot more properties (Required Services, Can Pause and Continue, Can Shutdown, Can Stop, etc.) of the Windows Service as shown on the screenshot below.
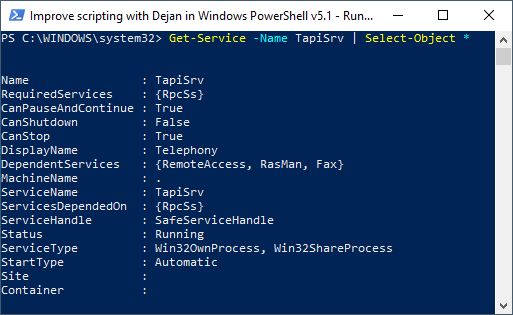
Notice that PowerShell version 6 and 7 will give us Description property which is not the case for some strange reason for PowerShell 5.1 and older as we can see on the screenshot below.
UPDATE: I have found the reason for the missing description and explained that in the article “Write Own Powershell CmdLet That Handles Windows Services” so please read the explanation there
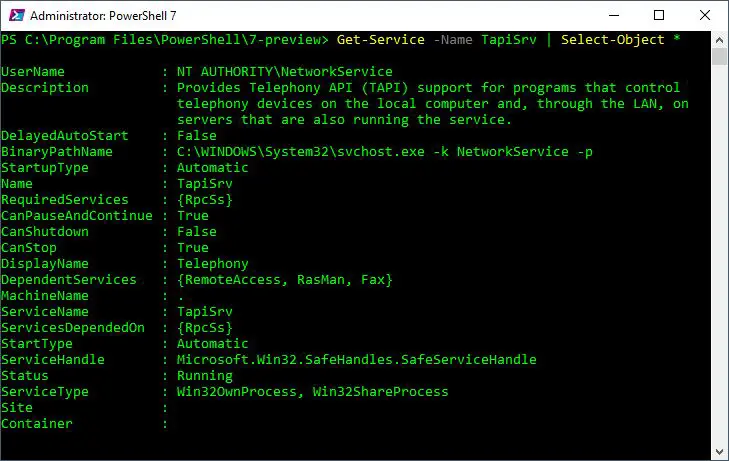
- We can use CIM Class CIM_Service and Get-CimInstance PowerShell CmdLet to get Windows Service Properties running the following line of code:
Get-CimInstance -ClassName CIM_Service -Filter "Name='TapiSrv'" | Select-Object *
NOTE: It took a significantly longer time on my machine to get the result using this example comparing with other approaches so be aware of potential performance costs while deciding which approach to implement.
INFO: If you want to measure the performance of your code please read the article “How To Benchmark Scripts With PowerShell” where you can get data on how long takes to run your code.
Here is the result of running the above line of code. (The same result is in PowerShell versions 6 and 7).

- We can use WMI Class Win32_Service and Get-CimInstance PowerShell CmdLet to get Windows Service Properties running the following line of code:
Get-CimInstance -ClassName win32_service -Filter "Name='TapiSrv'" | Select-Object *
Here is the result of running the above line of code. (The same result is in PowerShell versions 6 and 7).
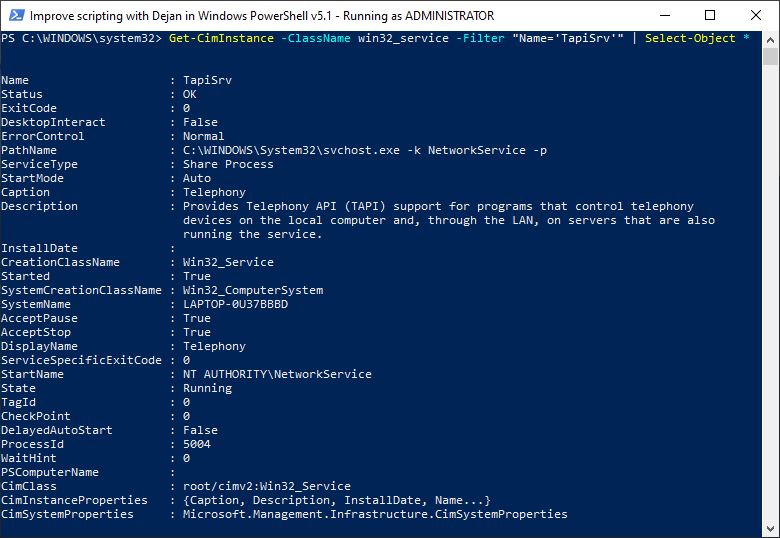
- Finally, we can use WMI Class Win32_Service but this time with Get-WmiObject PowerShell CmdLet to get Windows Service Properties running the following line of code:
Get-WmiObject -ClassName win32_service -Filter "Name='TapiSrv'" | Select-Object *
NOTE: This call will work on PowerShell version 5.1 and older but not on PowerShell version 6 and 7 since Get-WmiObject CmdLet has been retired as we can see on the screenshots below.
Here is the result of running the above line of code in PowerShell version 5.1
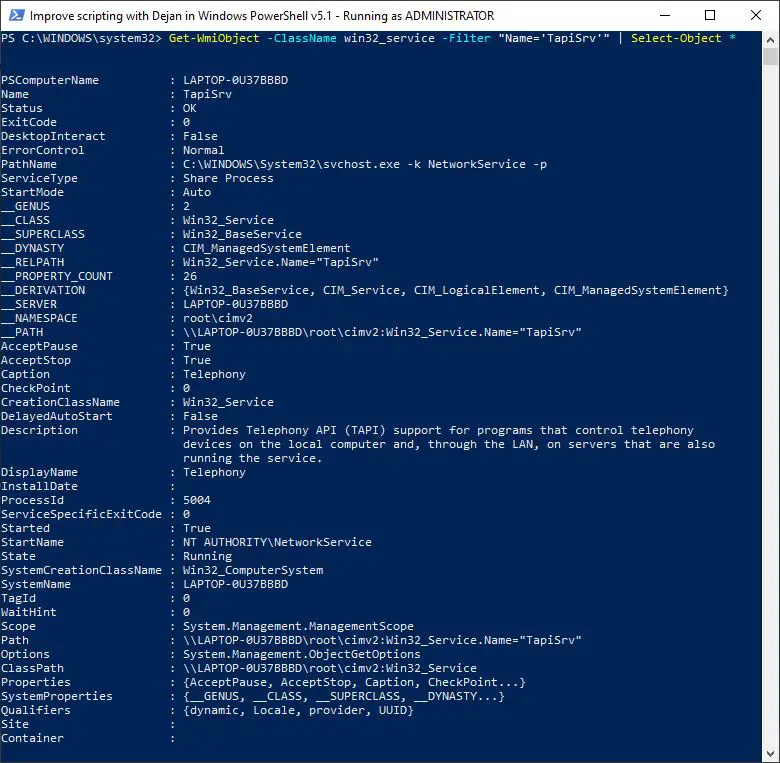
Here is the error code if we try to run the same line of code in PowerShell Version 6 and as we can see the Get-WmiObject CmdLet has not been recognized.

The error code is very similar on PowerShell version 7 but the suggestion is a little bit confusing as you can see on the screenshot below.
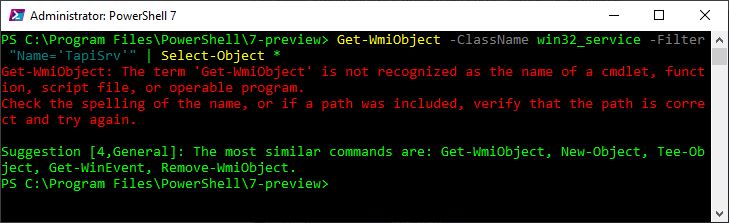
INFO: If you want to explore CIM or WMI Classes I have written two articles that go more in detail about how to get all the properties of certain CIM or WMI Classes.
How To List CIM Or WMI Class All Properties And Their Datatypes With PowerShell
How To Write Select Statement For All Properties Of CIM Or WMI Class With PowerShell
How To Get Windows Service Properties On Remote Computers Using PowerShell With Easy Steps
In order to check Windows Service Properties on Remote Computers, we will use Invoke-Command PowerShell CmdLet that demands several features enabled and configured which I will explain in the next subtopic and refer to it in other examples that use remoting throughout this article.
How To Configure PowerShell Remoting And Credentials
Here are guidance how to enable remoting on the servers against which the sample codes will run.
Since we will use Invoke-Command PowerShell CmdLet we need need to enable remoting using Enable-PSRemoting CmdLet and that will configure WinRM Windows Service used by the PowerShell Remoting technology.
For my environment, I have used Stand-Alone installation and that means everything runs on the same machine so you need to research which installation and configuration are appropriate for your environment.
INFO: How To Enable Remoting is out of the scope of this article since we have so many different environments and scenarios to cover up. Please read more in the Microsoft article Enable-PSRemoting and About Remote in order to learn more about PowerShell Remoting.
Hand in hand with remoting we need to think about the user that will run the code and the user’s credentials. Again configuration of credentials depends on the environments and you need to research and apply appropriate configuration for your environment.
Here I will explain the steps applied for my Stand-Alone Remoting environment in order to have the necessary credentials.
- First, we will save the user’s password in an external encrypted text file using the following code
Read-Host -AsSecureString | ConvertFrom-SecureString |
Out-File -FilePath "C:\Users\dekib\Documents\PSCredential\Invoke-Command.txt"
- When we run the code we will be prompted to enter the user’s password that will be encrypted and saved in an external text file as you can see on the screenshot below.
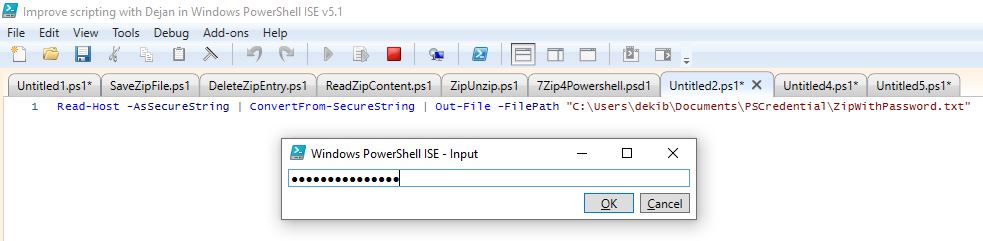
- Now we can reuse saved password whenever we need as we can see in the example below that will be used in all remote computers examples in this article.
$EncryptedPasswordFile = "C:\Users\user_name\Documents\PSCredential\Invoke-Command.txt"
$username="user_name"
$password = Get-Content -Path $EncryptedPasswordFile | ConvertTo-SecureString
$Credentials = New-Object System.Management.Automation.PSCredential($username, $password)
NOTE: I have stand-alone enabled Remoting. That means everything has been enabled and configured on the same computer. For that reason, I have used this approach of keeping my credentials. You have to find out which solution is right for your environment.
Now we are ready to show the example.
Example – How To Get Windows Service Properties On Remote Computer Using PowerShell With Easy Steps
When we have gotten out of the way PowerShell Remoting and User Credentials we can focus on our example following these steps.
- Open Windows PowerShell ISE or Visual Studio Code as Administrator
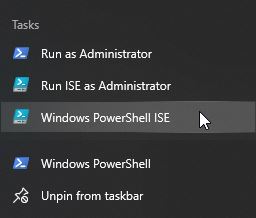
- Run the following lines of code in order to check Windows Service Properties on the Remote Servers.
##REPLACE THIS VALUE!!
$EncryptedPasswordFile = "C:\Users\dekib\Documents\PSCredential\Invoke-Command.txt"
##REPLACE THIS VALUE!!
$username="user_name"
$password = Get-Content -Path $EncryptedPasswordFile | ConvertTo-SecureString
$Credentials = New-Object System.Management.Automation.PSCredential($username, $password)
##REPLACE THIS VALUE!!
$computers = Get-Content C:\Temp\OKFINservers.txt
$scriptblock = { Get-Service -Name TapiSrv }
Invoke-Command -ComputerName $computers -Credential $Credentials -ScriptBlock $scriptblock
Let me quickly explain the above code line by line.
Code Explanation – Line By Line
First, we ready the value of the encrypted user’s password saved in an external text file and save in variable $EncryptedPasswordFile. To learn how to save user’s credentials please read the subheading “How To Configure PowerShell Remoting And Credentials“. Please point to the correct location in your code in order for this to work for you.
Second, we save the user’s name in the variable $username. Again, please replace this value with your user’s name in order for the code to work for you.
Third, we read the content of the encrypted text file where the user’s password has been stored and PowerShell Pipeline to ConvertTo-SecureString PowerShell CmdLet in order that variable $password holds the value in the correct format.
Fourth, we create a user credential object using New-Object PowerShell CmdLet providing the user’s name and password values.
Fifth, we get the list of remote computers from the external text file and save in variable $computers. Please point to the correct file path in your example in order for your code to work.
Here is the content of the text file with the list of servers in my example. I have used the same server for all the “remote” computers but you will replace this with the names of your servers.
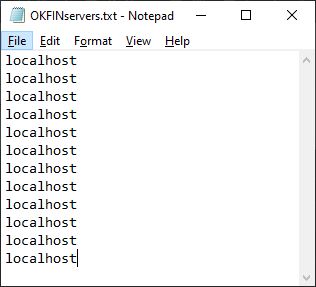
Sixth, we save the call to Get-Service PowerShell CmdLet in variable $scriptblock which will run on each of the remote servers and collect information about that Windows Service.
Finally, we can call Invoke-Command PowerShell CmdLet and provide the values for each needed parameter (Computer Name, Credentials, and Script Block)
Here is the result of running the code in Windows PowerShell ISE.
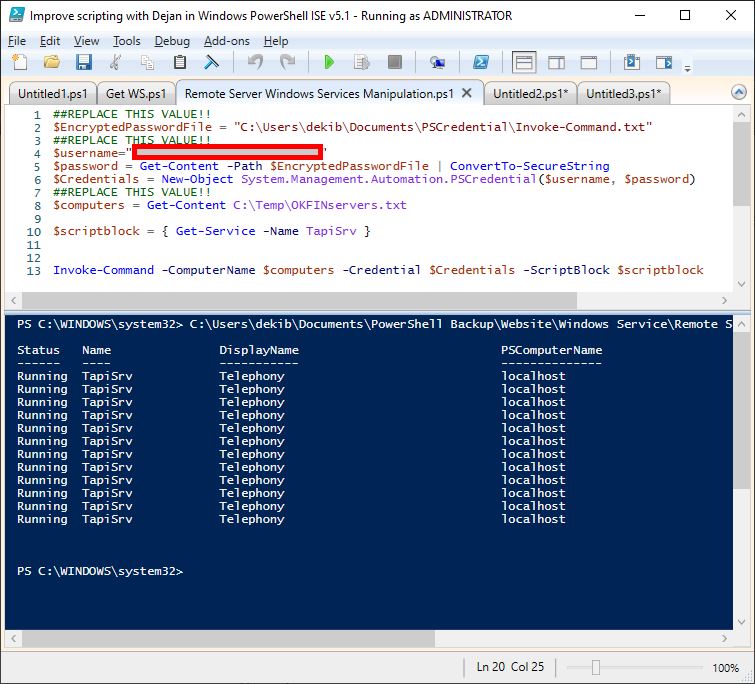
Here is the result of running the same code in Visual Studio Code.
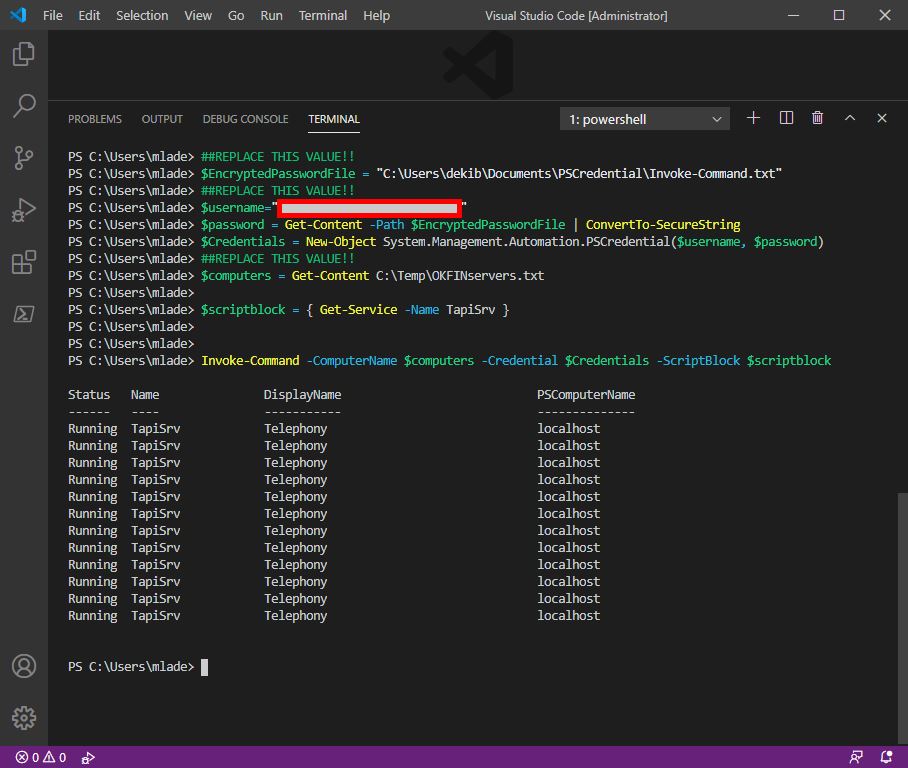
How To Start Windows Service Using PowerShell With Easy Steps
In order to start Windows Service using PowerShell we will exploit Start-Service PowerShell CmdLet using the following steps:
- Open PowerShell Console running as Administrator
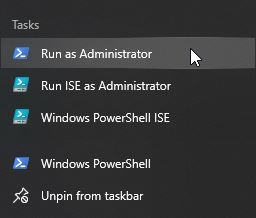
- Run the following line of code in order to start the Telephony Windows Service using PowerShell Console:
Start-Service -Name TapiSrv
REMEMBER: Change the value for the Name parameter to the name of your windows service that you want to start.
- Check that Telephony Windows Service is now running by running the following command in PowerShell Console:
Get-Service -Name TapiSrv
Here is the result and the proof that Telephony Windows Service is running again.

The telephony windows service was first stopped before running the command as you can see on the screenshot below.
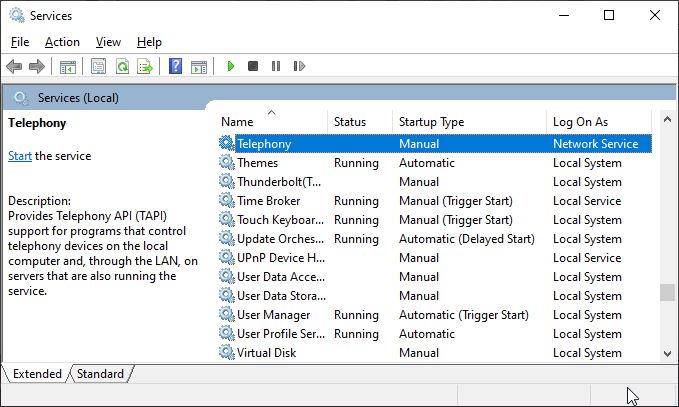
After running the Start-Service command Telephony windows service has been started and running again as you can see on the screenshot below.

As a bonus, we can see one example where we can combine both starting of the windows service and giving the feedback about the status of windows service so we avoid two lines of code as we had in the above example.
Start-Service -Name TapiSrv -PassThru | Select-Object *
We use the PassThru parameter that will send down the Powershell Pipeline windows service object so we can use Select-Object CmdLet to present the properties of the Windows Service to the user.
Let me discuss a few nuances of the Start-Service PowerShell CmdLet and what we need to be aware of in order to have expected results from running this CmdLet instead of some nasty surprises.
NOTE: We need to be aware of the following features of Start-Service CmdLet:
– The user running the Start-Service CmdLet needs to have enough permissions to start service in Windows. That is the reason why is the best option to start PowerShell Console as Administrator.
– We can start only windows services with start type of Manual, Automatic, or Automatic (Delayed) but not Disabled windows services. Look at the code example below of how to handle this scenario.
How To Start Disabled Windows Service Using PowerShell With Easy Steps
If the Windows Service has been disabled we cannot start it without first changing Start Type to the “Manual” value and then starting the Windows service as we show in the following steps.
- Open PowerShell Console running as Administrator
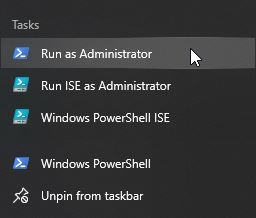
- This step is completely optional but we can first just check the Windows Service start type and whether it is disabled or not by running the following line of code
Get-Service -Name TapiSrv | Select-Object -Property StartType
As we can see on the screenshot below the Telephone Windows Services has been disabled.
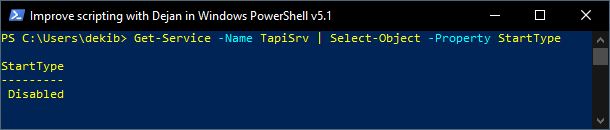
- In order to be able to start the disabled windows service, we need to change the start type to manual first using Set-Service PowerShell CmdLet.
Set-Service -Name TapiSrv -StartupType Manual
or even better we can set the windows service start type to manual and see that property value has actually changed using the following one-line code:
Set-Service -Name TapiSrv -StartupType Manual -PassThru | Select-Object -Property StartType
Here is the result of the last code example which shows us the changed start type to Manual

- We can start the windows service running the following line of code using Start-Service PowerShell CmdLet:
Start-Service -Name TapiSrv
- Finally, we can check that Windows Service has been started using Get-Service CmdLet.
Get-Service -Name TapiSrv
As we can see on the screenshot Telephony Windows Service has been started after being initially disabled.
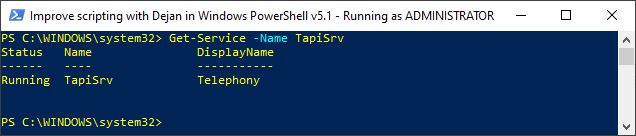
How To Start Windows Service On Remote Computers Using PowerShell With Easy Steps
If we want to start Windows Service on the Remote Computers we can use Invoke-Command PowerShell CmdLet and PowerShell Remoting.
I have already explained my configuration of PowerShell Remoting in the subtopic “How To Configure PowerShell Remoting And Credentials“, so please read this first if you need to know more about that and come back to continue with this example.
- Open Windows PowerShell ISE as Administrator
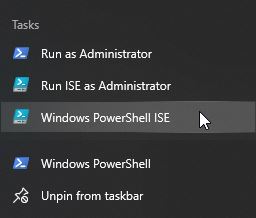
- Run the following lines of code in order to Start Windows Service on Remote Computers.
##REPLACE THIS VALUE!!
$EncryptedPasswordFile = "C:\Users\dekib\Documents\PSCredential\Invoke-Command.txt"
##REPLACE THIS VALUE!!
$username="user_name"
$password = Get-Content -Path $EncryptedPasswordFile | ConvertTo-SecureString
$Credentials = New-Object System.Management.Automation.PSCredential($username, $password)
##REPLACE THIS VALUE!!
$computers = Get-Content C:\Temp\OKFINservers.txt
$scriptblock = { Start-Service -Name TapiSrv -PassThru | Select-Object Name, Status }
Invoke-Command -ComputerName $computers -Credential $Credentials -ScriptBlock $scriptblock
I have already explained the code example line by line so please read that if needed on this link and come back to continue with this example.
The only difference is that we have in variable $scriptblock call to Start-Service PowerShell CmdLet and Pipeline result to Select-Object CmdLet in order to get the feedback on the status of the Windows Service.
Here is the result of running the above code.
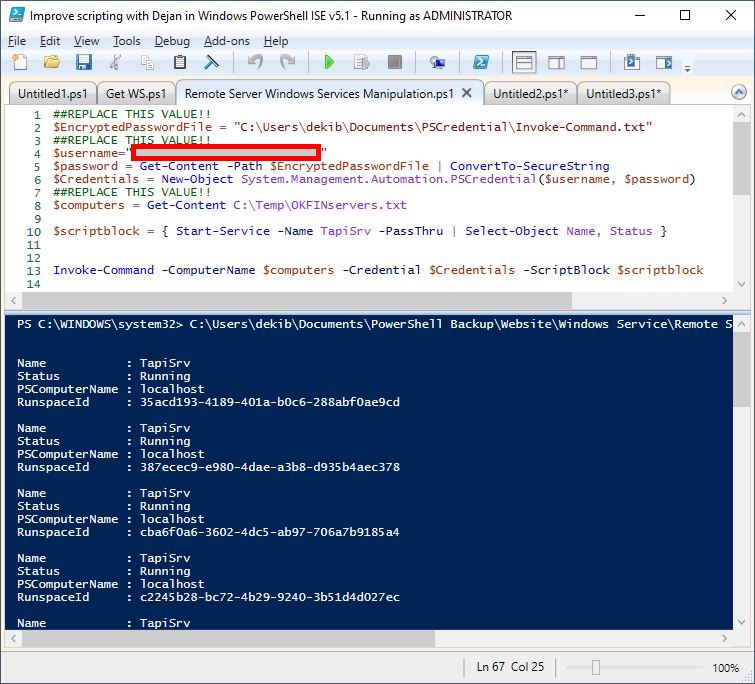
Start-Service PowerShell CmdLet Input Parameters Explained With Examples
In the previous examples we have already used some input parameters of Start-Service CmdLet but let’s look at the additional parameters and how we can use them and when is useful to implement them.
Here are the Start-Service CmdLet Input Parameters explained:
- The Name parameter is optional and specifies the windows service(s) name(s) that needs to be started and
- can be passed as a string array and,
- accepts pipeline input values,
- but we cannot use wildcards characters in the service names.
Here is one example where we implement the Name parameter of Start-Service CmdLet:
Start-Service -Name TapiSrv
- The DisplayName parameter is optional and specifies the display name of the windows service(s) and
- can be passed as a string array and,
- accepts wildcard characters in the display names which is an advantage comparing with the previous Name parameter
- but doesn’t accepts values from PowerShell Pipeline as we can do it with the Name parameter
Here is an example call of Start-Service CmdLet using the DisplayName Parameter, notice how we have used wild card which is not possible using the Name parameter:
Start-Service -DisplayName "Teleph*"
- The InputObject parameter specifies ServiceController objects which are nothing but windows services interpreted in the PowerShell way which is very useful combined with PowerShell Pipeline to manipulate Windows Service.
Here is an example of a call to Start-Service CmdLet and implementation of InputObject parameter:
Start-Service -InputObject (Get-Service -Name TapiSrv)
- The PassThru parameter returns an object that represents the windows service and it is very useful to get immediate feedback on the Start-Service CmdLet run outcome.
In this example, we use just one line of code instead of running Start-Service followed by Get-Service in order to get feedback on what happened with the status of Windows Service
Start-Service -Name TapiSrv -PassThru | Select-Object *
- The WhatIf parameter helps us to evaluate what will happen if we run the command without actually executing it. It is very useful for testing the code.
Here is one example of using Start-Service CmdLet with the WhatIf parameter implemented:
Start-Service -DisplayName "Teleph*" -WhatIf
- The Confirm parameter prompts the user to confirm the execution of the code before actually running it.
Start-Service -DisplayName "Teleph*" -Confirm
- The Include parameter specifies an array of strings that represent Windows Services Names that will be included to start the windows services.
We want to start all the Windows Services with a Name pattern starting as “app*” but include only two of these windows services with specified names (AppIDSvc, AppReadiness).
Start-Service -Name "app*" -Include AppIDSvc, AppReadiness
- The Exclude parameter specifies an array of strings that represent Windows Services Names and Start-Service CmdLet will omit these names while trying to run the other services with a similar name. It is useful for starting a batch of services with some exceptions in the pattern.
In this example, Start-Service will start all the windows services whose name starts with “app*” but will omit Appinfo Windows service to start.
Start-Service -Name "app*" -Exclude Appinfo
How To Stop Windows Service Using PowerShell With Easy Steps
We will use Stop-Service PowerShell CmdLet in order to stop the Windows Service following these steps:
- Open PowerShell Console running as Administrator
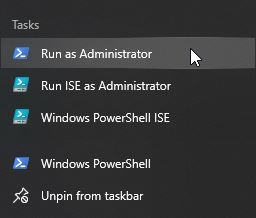
- Run the following line of code in order to Stop the Adobe Genuine Monitor Service Windows Service using PowerShell Console:
Stop-Service -Name AGMService
REMEMBER: Change the value for the Name parameter to the name of your windows service that you want to stop.
NOTE: Adobe Genuine Monitor Service Windows Service doesn’t depend on any other windows service otherwise we need to use the Force parameter in addition when trying to stop windows services with dependencies which we will see in our next example. Read “How To Know Dependent Windows Services Using PowerShell” to find dependant services.
- Check that Adobe Genuine Monitor Service Windows Service has been stopped now by running the following command in PowerShell Console:
Get-Service -Name AGMService
On the screenshot below we can see that Adobe Genuine Monitor Service Windows Service has been stopped
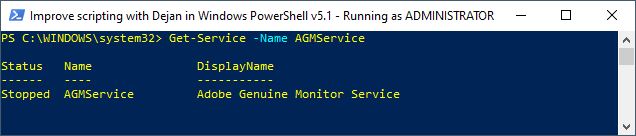
The same result we can see using Services Console.
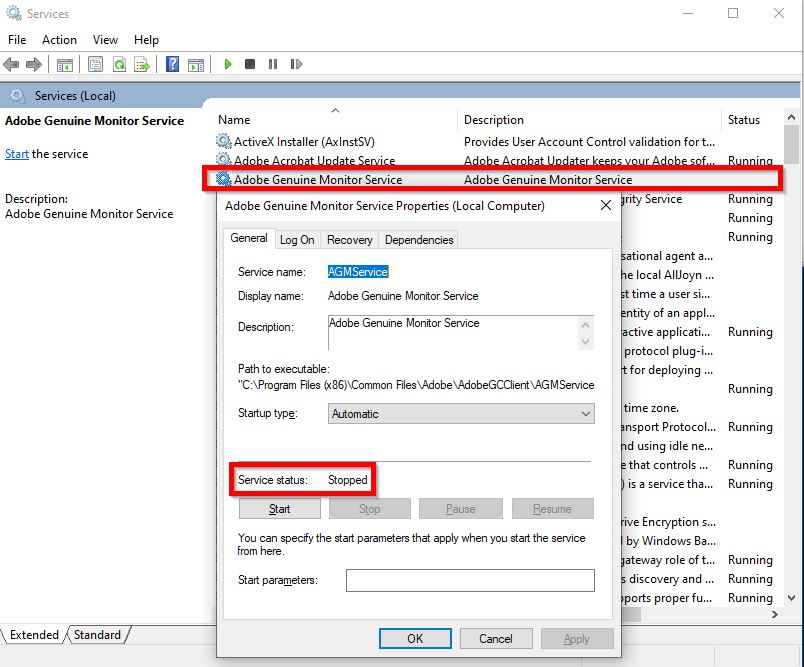
Let me discuss a few nuances of the Stop-Service PowerShell CmdLet and what we need to be aware of in order to have expected results from running this CmdLet instead of some nasty surprises.
NOTE: We need to be aware of the following features of Stop-Service CmdLet:
– The user running the Stop-Service CmdLet needs to have enough permissions to stop service in Windows. That is the reason why is the best option to start PowerShell Console as Administrator.
– Use the Force parameter if windows service has dependant service(s) in order to stop both wanted windows service and dependant service(s).
How To Stop Windows Service That Has Dependent Services Using PowerShell
When the Windows Services has dependent windows services we need to implement the Force parameter when we run Stop-Service PowerShell CmdLet so both dependant and main windows services have been stopped.
Let’s explore this example.
- Open PowerShell Console running as Administrator
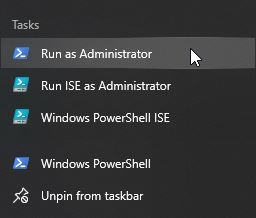
- Let’s for the sake of experimenting trying to stop windows service without using the Force parameter running the following line of code:
Stop-Service -Name TapiSrv
Since Telephony Windows Service depends on some other windows services we get the error while trying to stop the service without implementing the Force parameter as you can see on the screenshot.
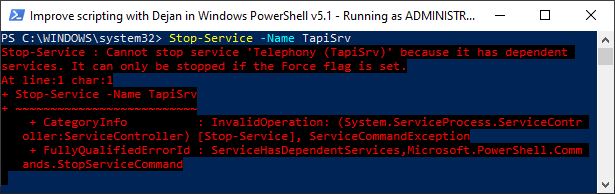
- If we run the same line of code but this time in addition we implement the Force parameter the outcome will be different.
Stop-Service -Name TapiSrv -Force
- The last thing is to check with Get-Service CmdLet if windows service has been actually stopped.
Get-Service -Name TapiSrv
The outcome can be seen on the following screenshot.
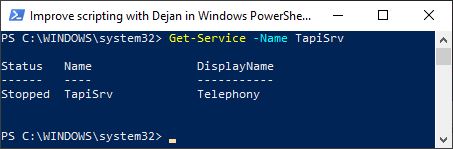
- Or we can accomplish all of this in just one line of code without using Get-Service CmdLet if we implement PassThru parameter and combine the result with PowerShell Pipeline and Select-Object CmdLet
Stop-Service -Name TapiSrv -Force -PassThru | Select-Object *
Here is the result of the above code and we can see that the windows service has been stopped.
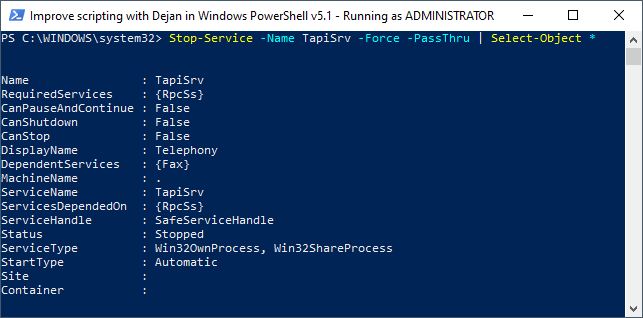
How To Know Dependent Windows Services Using PowerShell
If we want to know dependent windows service(s) for the windows service we can run the following line of code.
Get-Service -Name TapiSrv -DependentServices
Here is the result of above code.
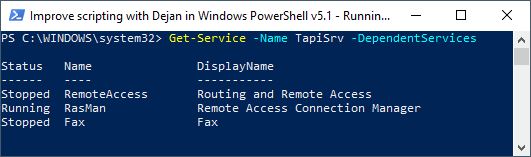
How To Know Required Windows Services Using PowerShell
If we want to know the required windows services for the windows service we can run the following line of code.
Get-Service -Name TapiSrv -RequiredServices
Here is the result of above code.
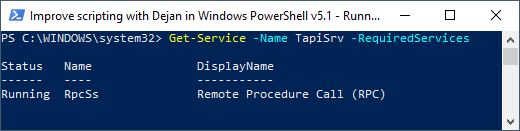
How To Stop Windows Service On Remote Computers Using PowerShell With Easy Steps
If we want to stop Windows Service on the Remote Computers we can use Invoke-Command PowerShell CmdLet and PowerShell Remoting.
I have already explained my configuration of PowerShell Remoting in the subtopic “How To Configure PowerShell Remoting And Credentials“, so please read this first if you need to know more about that and come back to continue with this example.
- Open Windows PowerShell ISE as Administrator
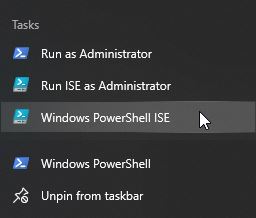
- Run the following lines of code in order to Stop Windows Service on Remote Computers.
##REPLACE THIS VALUE!!
$EncryptedPasswordFile = "C:\Users\dekib\Documents\PSCredential\Invoke-Command.txt"
##REPLACE THIS VALUE!!
$username="user_name"
$password = Get-Content -Path $EncryptedPasswordFile | ConvertTo-SecureString
$Credentials = New-Object System.Management.Automation.PSCredential($username, $password)
##REPLACE THIS VALUE!!
$computers = Get-Content C:\Temp\OKFINservers.txt
$scriptblock = { Stop-Service -Name TapiSrv -Force -PassThru | Select-Object Name, Status }
Invoke-Command -ComputerName $computers -Credential $Credentials -ScriptBlock $scriptblock
I have already explained the code example line by line so please read that if needed on this link and come back to continue with this example.
The only difference is that we have in variable $scriptblock call to Stop-Service PowerShell CmdLet and Pipeline result to Select-Object CmdLet in order to get the feedback on the status of the Windows Service.
Here is the result of running the above code.

How To Restart Windows Service Using PowerShell With Easy Steps
If we want to restart the Windows Service we can use native Restart-Service PowerShell CmdLet following these steps:
- Open PowerShell Console running as Administrator
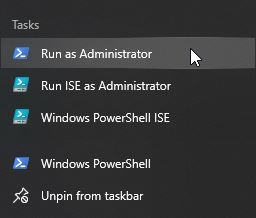
- Run Restart-Service CmdLet code below in order to restart the windows service:
Restart-Service -Name TapiSrv -Force
- It is always smart to check the status of the Windows Service that we have just restarted running the following piece of code:
Get-Service -Name TapiSrv | Select-Object *
On the screenshot we can see the result of running above line of code.

NOTE: It is very important to run Restart-Service CmdLet code with a user who has enough privileges to manipulate Windows Services and for that reason, it is smart to run PowerShell Console as Administrator.
As a bonus, we can see one example where we can combine both restarting of the windows service and giving feedback about the status of windows service so we avoid two lines of code as we had in the above example.
Restart-Service -Name TapiSrv -Force -PassThru | Select-Object *
We use the PassThru parameter that will send down the Powershell Pipeline windows service object so we can use Select-Object CmdLet to present the properties of the Windows Service to the user.
NOTE: We used the Force parameter to restart the dependant service (RasMan – Remote Access Connection Manager) as well. Read “How To Know Dependent Windows Services Using PowerShell” to find dependant services.
Here is the result of the above line of code and we can see in the status column that the windows service has been started after the restart.
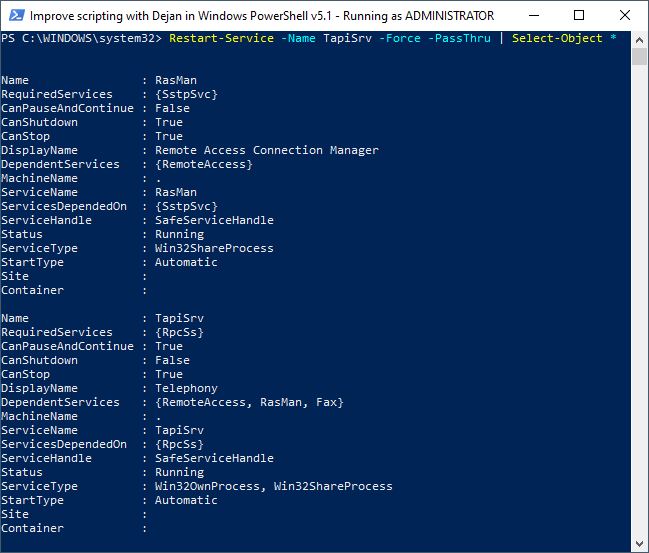
How To Restart Windows Service On Remote Computers Using PowerShell With Easy Steps
If we want to restart Windows Service on the Remote Computers we can use Invoke-Command PowerShell CmdLet and PowerShell Remoting.
I have already explained my configuration of PowerShell Remoting in the subtopic “How To Configure PowerShell Remoting And Credentials“, so please read this first if you need to know more about that and come back to continue with this example.
- Open Windows PowerShell ISE as Administrator
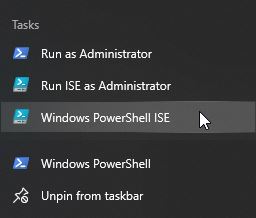
- Run the following lines of code in order to Restart Windows Service on Remote Computers.
##REPLACE THIS VALUE!!
$EncryptedPasswordFile = "C:\Users\dekib\Documents\PSCredential\Invoke-Command.txt"
##REPLACE THIS VALUE!!
$username="user_name"
$password = Get-Content -Path $EncryptedPasswordFile | ConvertTo-SecureString
$Credentials = New-Object System.Management.Automation.PSCredential($username, $password)
##REPLACE THIS VALUE!!
$computers = Get-Content C:\Temp\OKFINservers.txt
$scriptblock = { Restart-Service -Name TapiSrv -Force -PassThru | Select-Object Name, Status }
Invoke-Command -ComputerName $computers -Credential $Credentials -ScriptBlock $scriptblock
I have already explained the code example line by line so please read that if needed on this link and come back to continue with this example.
The only difference is that we have in variable $scriptblock call to Restart-Service PowerShell CmdLet and Pipeline result to Select-Object CmdLet in order to get the feedback on the status of the Windows Service.
Here is the result of running the above code.
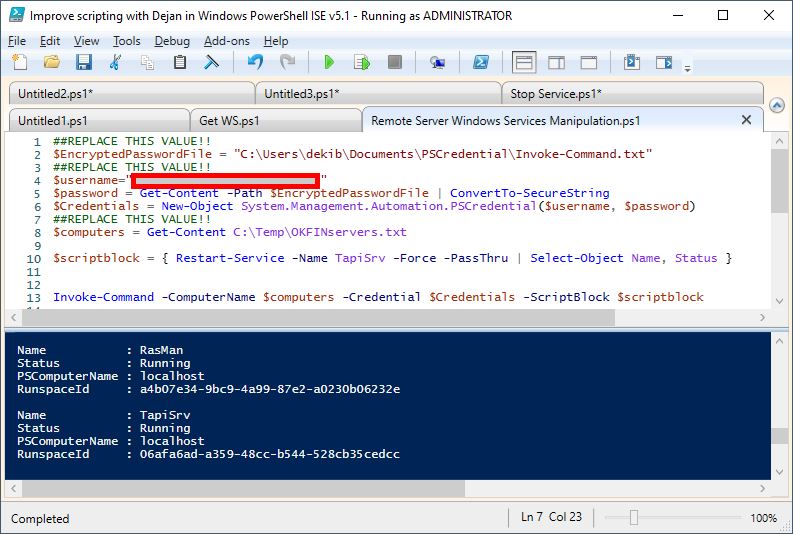
How To Pause (Suspend) Windows Service Using PowerShell With Easy Steps
When we just want to pause (suspend) some Windows Service we can use native Suspend-Service PowerShell CmdLet using the following steps:
- Open PowerShell Console running as Administrator
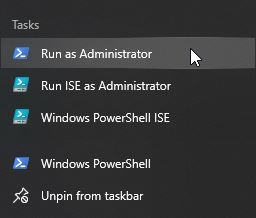
- Run Suspend-Service CmdLet code below in order to pause (suspend) the windows service:
Suspend-Service -Name TapiSrv
- We can check the status of the Windows Service to confirm that Windows Service has been actually paused (suspended):
Get-Service -Name TapiSrv
Here is the result of running the previous line of code and please pay attention to the status property value that is now “Paused”
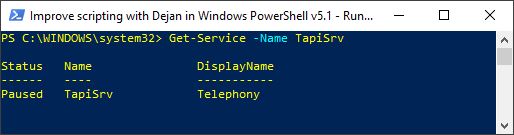
The same thing we can see in the Services Console and again notice the value in the Status column.
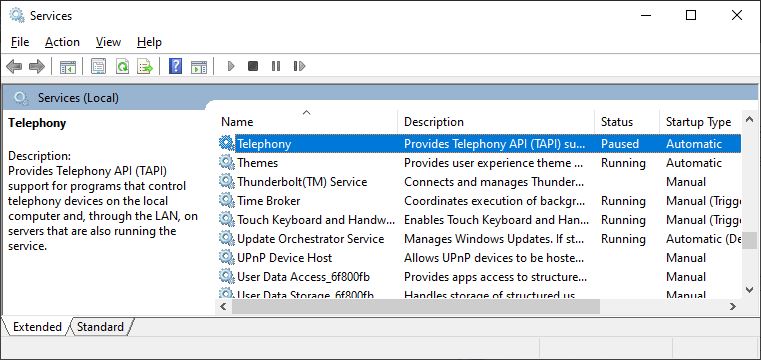
NOTE: It is very important to run Suspend-Service CmdLet code with a user who has enough privileges to manipulate Windows Services and for that reason, it is smart to run PowerShell Console as Administrator.
As a bonus, we can see one example where we can combine both pausing (suspending) the windows service and giving feedback about a change of windows service status so we avoid two lines of code as we had in the above example.
Suspend-Service -Name TapiSrv -PassThru | Select-Object *
We use the PassThru parameter that will send down the Powershell Pipeline windows service object so we can use Select-Object CmdLet to present the properties of the Windows Service to the user.
Here is the result of the above line of code and we can see in the status column that the windows service has been paused.
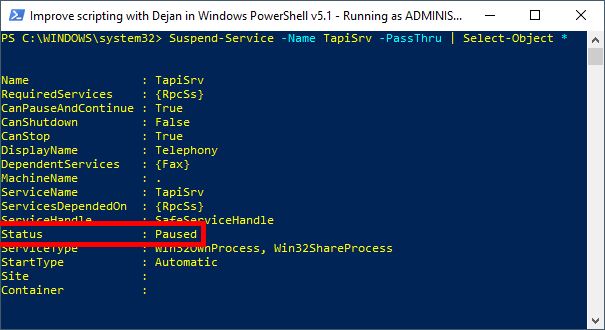
Which Windows Services Can Be Paused (Suspended) And Resumed Using PowerShell
REMEMBER: Not all Windows Services can be paused (suspended) and in order to check which Windows Services can or cannot be paused and resumed please use the following example code.
We use Get-Service PowerShell CmdLet to list all the Windows Services on the system and that result we PowerShell Pipeline to the Where-Object PowerShell CmdLet which is used to filter out only Windows Services that can be paused and resumed further down the PowerShell Pipeline we pass that result to Select-Object PowerShell CmdLet just to pick properties that we want to present to the user and finally, we use Sort-Object to sort the Windows Services by Display Name.
Get-Service | Where-Object {$_.CanPauseAndContinue -eq $true} | Select-Object DisplayName, Name, CanPauseAndContinue | Sort-Object DisplayName
Here is the result of the previous line of code:
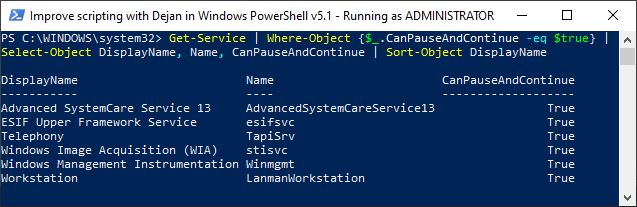
How To Pause (Suspend) Windows Service On Remote Computers Using PowerShell With Easy Steps
If we want to pause (suspend) Windows Service on the Remote Computers we can use Invoke-Command PowerShell CmdLet and PowerShell Remoting.
I have already explained my configuration of PowerShell Remoting in the subtopic “How To Configure PowerShell Remoting And Credentials“, so please read this first if you need to know more about that and come back to continue with this example.
- Open Windows PowerShell ISE as Administrator
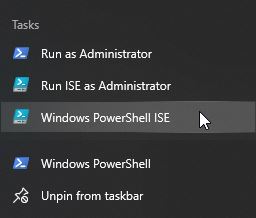
- Run the following lines of code in order to Pause (Suspend) Windows Service on Remote Computers.
##REPLACE THIS VALUE!!
$EncryptedPasswordFile = "C:\Users\dekib\Documents\PSCredential\Invoke-Command.txt"
##REPLACE THIS VALUE!!
$username="user_name"
$password = Get-Content -Path $EncryptedPasswordFile | ConvertTo-SecureString
$Credentials = New-Object System.Management.Automation.PSCredential($username, $password)
##REPLACE THIS VALUE!!
$computers = Get-Content C:\Temp\OKFINservers.txt
$scriptblock = { Suspend-Service -Name TapiSrv -PassThru | Select-Object Name, Status }
Invoke-Command -ComputerName $computers -Credential $Credentials -ScriptBlock $scriptblock
I have already explained the code example line by line so please read that if needed on this link and come back to continue with this example.
The only difference is that we have in variable $scriptblock call to Suspend-Service PowerShell CmdLet and Pipeline result to Select-Object CmdLet in order to get the feedback on the status of the Windows Service.
Here is the result of running the above code.
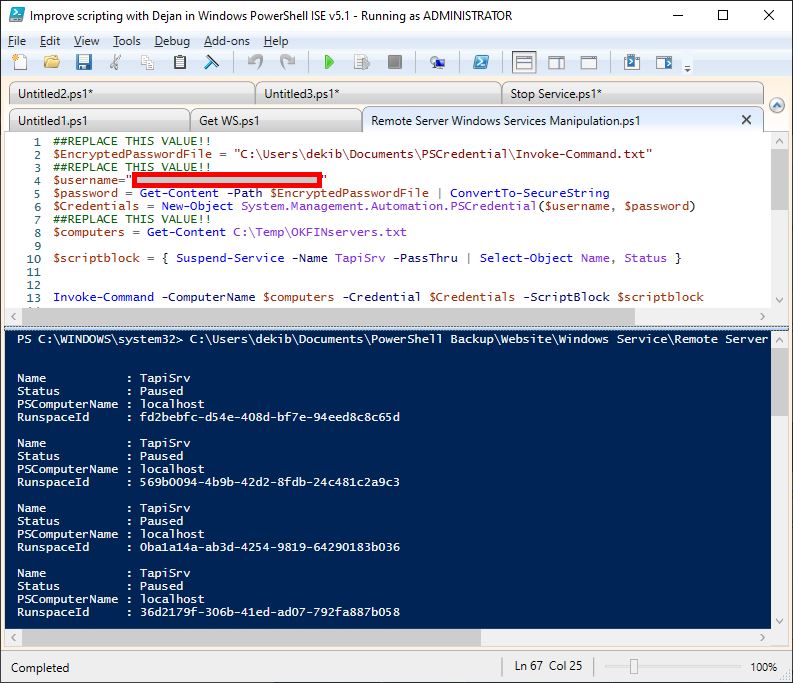
How To Resume Windows Service Using PowerShell With Easy Steps
To Resume Windows Service that has been suspended (paused) we can use Resume-Service PowerShell CmdLet following these steps:
- Open PowerShell Console running as Administrator
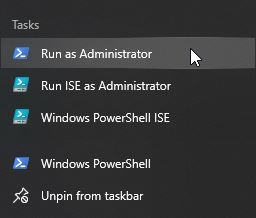
- Run Resume-Service CmdLet code below in order to resume the windows service that has been previously paused (suspended):
Resume-Service -Name TapiSrv
- We need to check if Windows Service has been started again using Get-Service PowerShell CmdLet
Get-Service -Name TapiSrv
Here is the result of running previous line of code and we can see in the status column that the Windows Services has been started again.
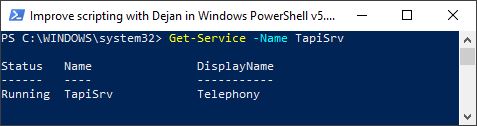
We can compare the state of the Windows Service before and after using Resume-Service CmdLet in Services Console as well.
In the first screenshot Telephony Windows Service has been paused and in the later screenshot Telephony Windows Service has been running after applying Resume-Service code.
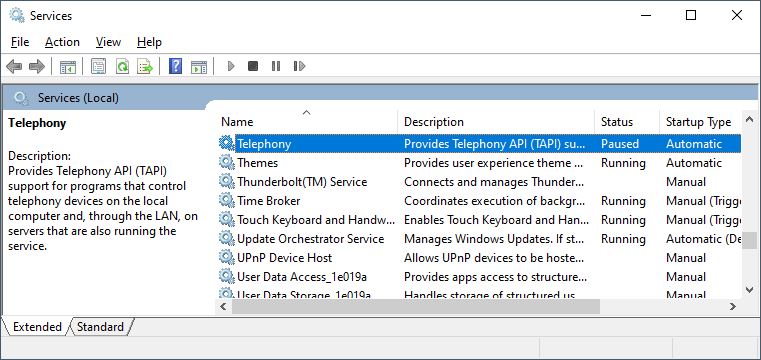
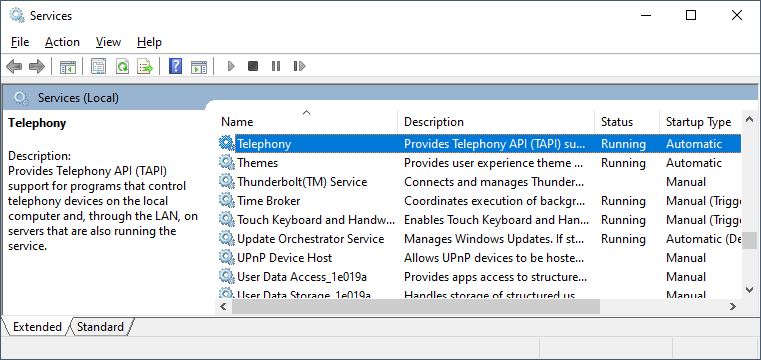
REMEMBER: Not all Windows Services can be paused (suspended) and in order to check which Windows Services can or cannot be paused and resumed please read the subheading “Which Windows Services Can Be Paused (Suspended) And Resumed Using PowerShell“.
As a bonus, we can see one example where we can combine both resuming (start again after a pause) the windows service and giving feedback about a change of windows service status so we avoid two lines of code as we had in the above example.
Resume-Service -Name TapiSrv -PassThru | Select-Object *
We use the PassThru parameter that will send down the Powershell Pipeline windows service object so we can use Select-Object CmdLet to present the properties of the Windows Service to the user.
Here is the result of the above line of code and we can see in the status column that the windows service has been started again after previously being paused (suspended).
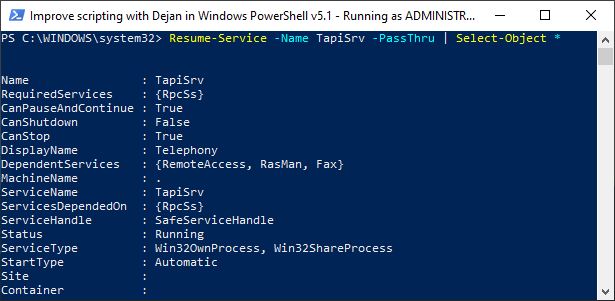
How To Resume Windows Service On Remote Computers Using PowerShell With Easy Steps
If we want to resume Windows Service on the Remote Computers we can use Invoke-Command PowerShell CmdLet and PowerShell Remoting.
I have already explained my configuration of PowerShell Remoting in the subtopic “How To Configure PowerShell Remoting And Credentials“, so please read this first if you need to know more about that and come back to continue with this example.
- Open Windows PowerShell ISE as Administrator
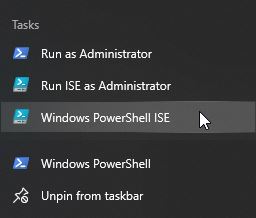
- Run the following lines of code in order to Resume Windows Service on Remote Computers.
##REPLACE THIS VALUE!!
$EncryptedPasswordFile = "C:\Users\dekib\Documents\PSCredential\Invoke-Command.txt"
##REPLACE THIS VALUE!!
$username="user_name"
$password = Get-Content -Path $EncryptedPasswordFile | ConvertTo-SecureString
$Credentials = New-Object System.Management.Automation.PSCredential($username, $password)
##REPLACE THIS VALUE!!
$computers = Get-Content C:\Temp\OKFINservers.txt
$scriptblock = { Resume-Service -Name TapiSrv -PassThru | Select-Object Name, Status }
Invoke-Command -ComputerName $computers -Credential $Credentials -ScriptBlock $scriptblock
I have already explained the code example line by line so please read that if needed on this link and come back to continue with this example.
The only difference is that we have in variable $scriptblock call to Resume-Service PowerShell CmdLet and Pipeline result to Select-Object CmdLet in order to get the feedback on the status of the Windows Service.
Here is the result of running the above code.
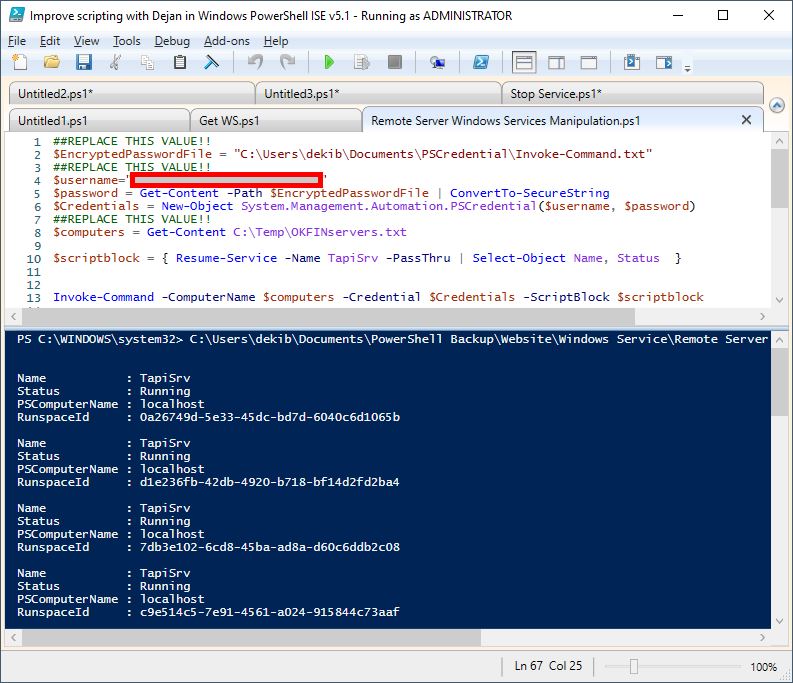
How To Change (Set) Windows Service Properties Using PowerShell With Easy Steps
When we want to Change (Set) Windows Service Properties we use Set-Service PowerShell CmdLet. Set-Service CmdLet is very powerful and can do many things such as change display name, the startup type of services, description, credentials. In addition, Set-Service CmdLet can start, stop, and suspend a windows service.
In this example, we will change Display Name, Description, and Startup Type of the ConDeamon Windows Service following these steps:
- Open PowerShell Console running as Administrator
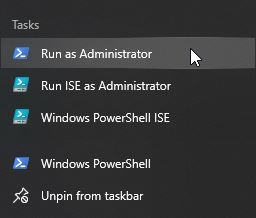
- Run Set-Service CmdLet code in order to change the properties of the Windows Service:
Set-Service -Name ConDaemon -DisplayName "New Con Deamon WS" -Description "Windows Service New Description" -StartupType Manual
- Now we need to check actual properties whether they have been changed to the new values running the following code.
Get-CimInstance -ClassName win32_service -Filter "Name='ConDaemon'" | Select-Object *
Here is the result of running the above code and we can see a change in the windows service properties (Display Name, Description, Startup Type)

We can compare the values of Windows Service Properties before and after changes in the Services Console as well. Notice the values for Name, Description, and Startup Type.
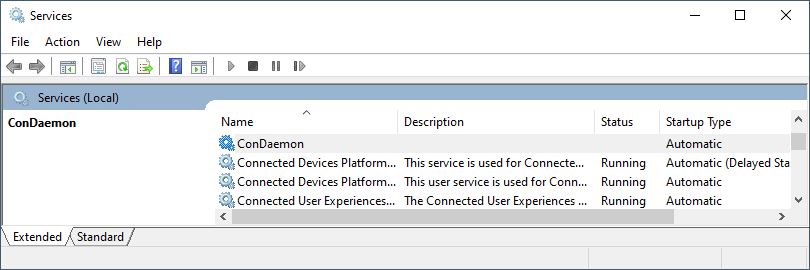
Here are new changed values for the Windows Service
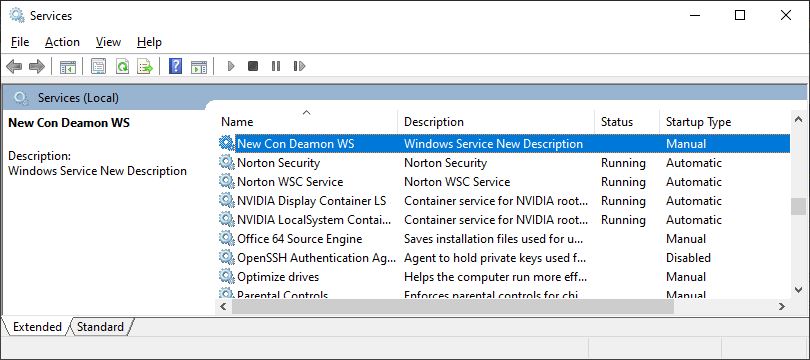
NOTE: We can set Windows Service Properties when we add it to the system if it is a new Windows Service as I have shown in “How To Add New Windows Service And Set Properties Using PowerShell With Easy Steps“
REMEMBER: Set-Service Windows Service can be very handy when we want to start disabled Windows Service as we have shown this in the “How To Start Disabled Windows Service Using PowerShell With Easy Steps“.
How To Change (Set) Windows Service Properties On Remote Computers Using PowerShell With Easy Steps
If we want to change (set) Windows Service on the Remote Computers we can use Invoke-Command PowerShell CmdLet and PowerShell Remoting.
I have already explained my configuration of PowerShell Remoting in the subtopic “How To Configure PowerShell Remoting And Credentials“, so please read this first if you need to know more about that and come back to continue with this example.
- Open Windows PowerShell ISE as Administrator
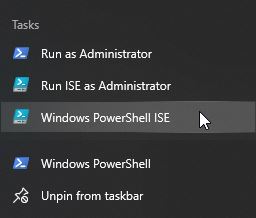
- Run the following lines of code in order to Set (Change) Windows Service on Remote Computers.
##REPLACE THIS VALUE!!
$EncryptedPasswordFile = "C:\Users\dekib\Documents\PSCredential\Invoke-Command.txt"
##REPLACE THIS VALUE!!
$username="user_name"
$password = Get-Content -Path $EncryptedPasswordFile | ConvertTo-SecureString
$Credentials = New-Object System.Management.Automation.PSCredential($username, $password)
##REPLACE THIS VALUE!!
$computers = Get-Content C:\Temp\OKFINservers.txt
$scriptblock = { Set-Service -Name ConDaemon -DisplayName "New Con Deamon WS" -StartupType Manual -PassThru | Select-Object Name, DisplayName, StartType, Status }
Invoke-Command -ComputerName $computers -Credential $Credentials -ScriptBlock $scriptblock
I have already explained the code example line by line so please read that if needed on this link and come back to continue with this example.
The only difference is that we have in variable $scriptblock call to Set-Service PowerShell CmdLet and Pipeline result to Select-Object CmdLet in order to get the feedback on the changed properties of the Windows Service.
Here is the result of running the above code.
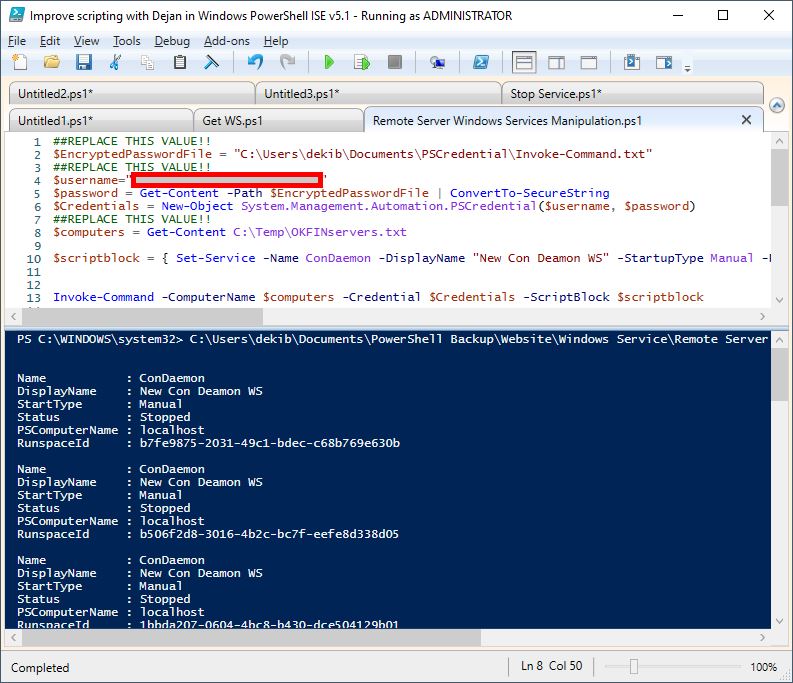
How To Add New Windows Service Using PowerShell With Easy Steps
If we want to add new windows service we will use New-Service PowerShell CmdLet by following these steps:
- Open PowerShell Console running as Administrator
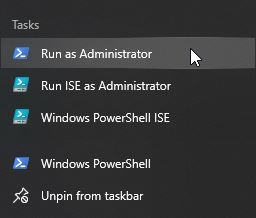
- Run New-Service CmdLet code in order to Add the ConDeamon Windows Service (Executable file located in the folder C:\Temp\WS) using PowerShell Console:
New-Service -Name "ConDaemon" -BinaryPathName "C:\Temp\WS\ConDaemon.exe"
Here is the result of above code after running it.

- Check that ConDeamon Windows Service has been added by using Get-Service PowerShell CmdLet:
Get-Service -Name ConDaemon | Select-Object *
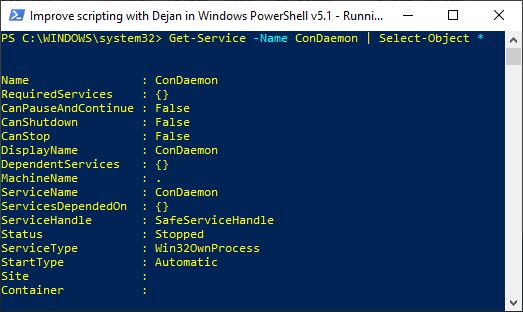
We can see ConDeamon Windows Service in the Services Console as well.
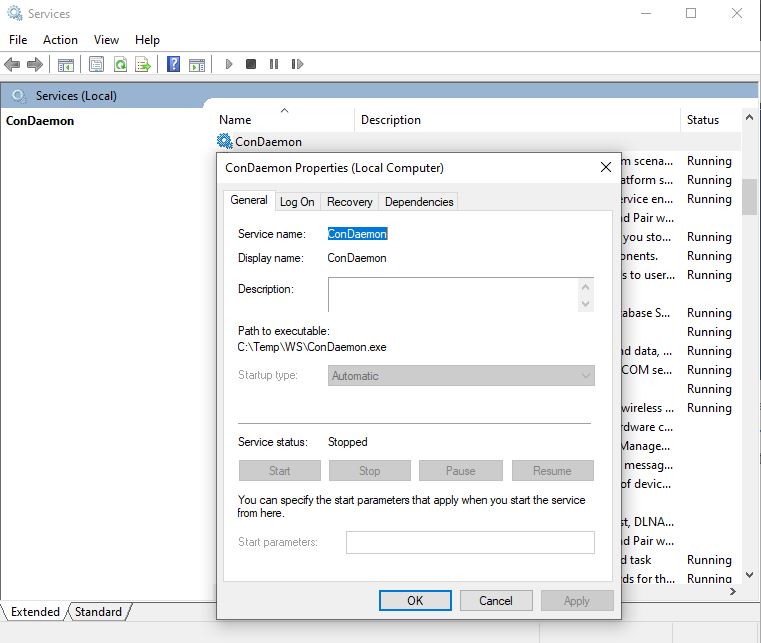
NOTE: Creating Windows Services is out of the scope of this article. ConDeamon Windows Service is part of some older tutorials that I have used in the past.
How To Add New Windows Service And Set Properties Using PowerShell With Easy Steps
Beside Adding the new Windows Service at the same time we can set windows service properties as well. Let me show how we can do that with several easy steps:
- Open PowerShell Console running as Administrator
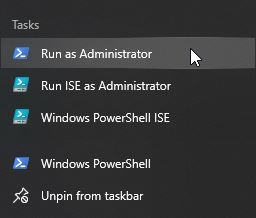
- Run New-Service CmdLet code in order to Add the ConDeamon Windows Service (Executable file located in the folder C:\Temp\WS) using PowerShell Console and at the same time we will set Display Name and Description of the windows service:
New-Service -Name "ConDaemon" -BinaryPathName "C:\Temp\WS\ConDaemon.exe" -DisplayName "Con Deamon Windows Service" -Description "Con Deamon Window Service is part of C# project Connection Manager"
Here is the result of the above code after running it.
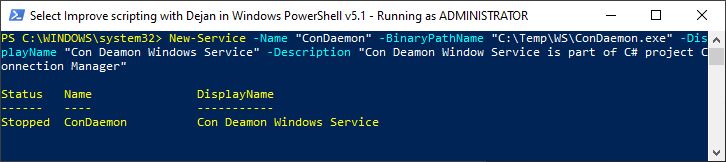
- Check that ConDeamon Windows Service has been added by using Get-Service PowerShell CmdLet:
Get-Service -Name ConDaemon | Select-Object *
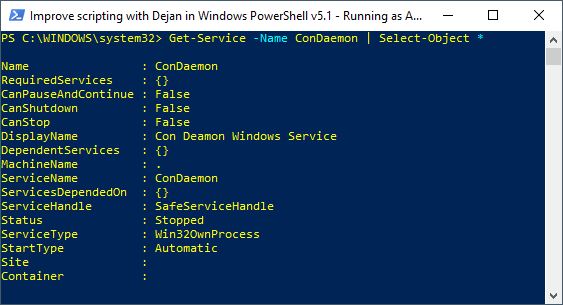
We can see ConDeamon Windows Service in the Services Console as well. Notice the values for Display Name and Description.
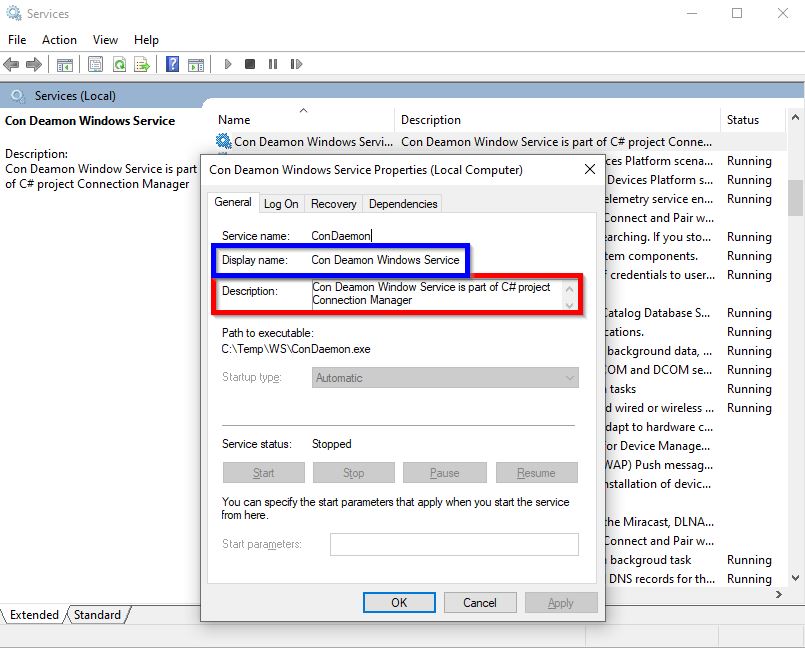
How To Delete (Remove) Windows Service Using PowerShell With Easy Steps
If we want to Delete (Remove) Windows Service we have several options but they depend on the PowerShell version that we are running with and we will cover them in this article.
How To Delete (Remove) Windows Service With PowerShell Version 5.1 And Older
First, let’s look at the solution for PowerShell version 5.1 and older. We will use the Delete Method of Win32_Service WMI Class using Get-WmiObject PowerShell CmdLet.
(Get-WmiObject -ClassName win32_service -Filter "Name='ConDaemon'").Delete()
Here is the screenshot with outcome of running the previous line of code:

If we check Services Console we cannot see ConDeamon Windows Service in the list of services as presented in the below screenshot.
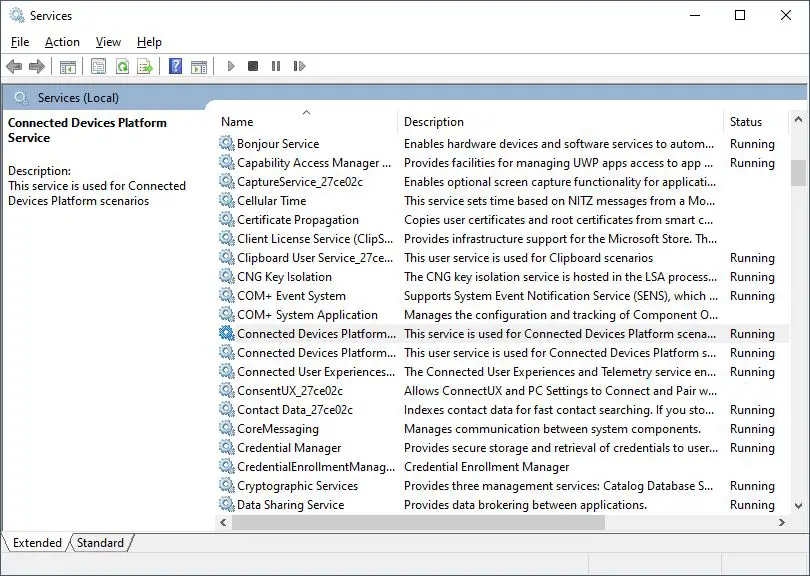
How To Delete (Remove) Windows Service With PowerShell Version 6 And Newer
For the PowerShell version 6.0 and above we have native Remove-Service PowerShell CmdLet that will remove (delete) the windows service so let’s look at the steps for that example:
- Open PowerShell Console running as Administrator

- Run Remove-Service CmdLet code below to remove (delete) Windows service:
Remove-Service -Name "ConDaemon"
or we can use this line of code as well with PowerShell Pipeline
Get-Service -Name ConDaemon | Remove-Service
In the Service Console we do not see anymore ConDeamon Windows Service.
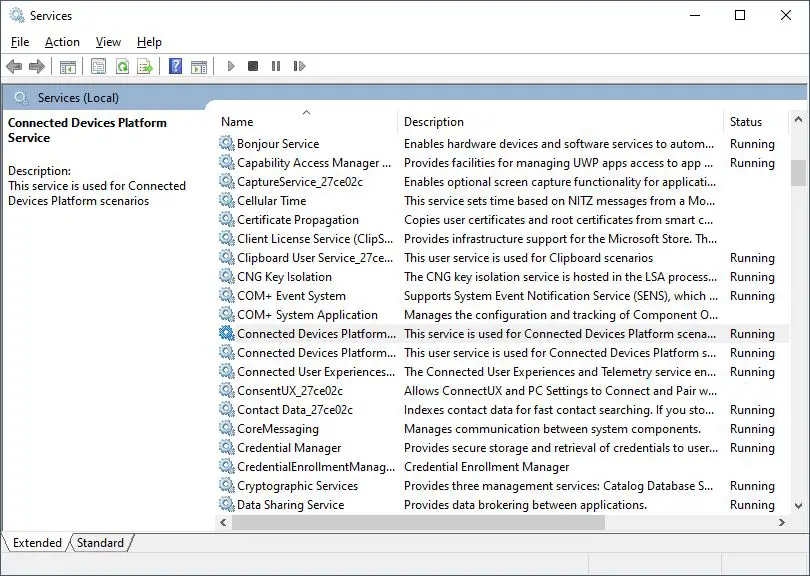